vue3中的数组
时间: 2023-07-03 18:06:12 浏览: 105
在Vue3中,数组的使用和Vue2基本上没有变化。你可以使用常规的JavaScript方法来操作数组,例如push、pop、shift、unshift、splice等。但是Vue3提供了一些新的API和语法糖来更方便地操作数组。
1. reactive API
Vue3中的reactive API可以让任何JavaScript对象变成响应式对象。这意味着,当对象的属性发生改变时,Vue会自动重新渲染相关的视图。这个API同样适用于数组,因此你可以使用它来创建响应式的数组。
```javascript
import { reactive } from 'vue'
const state = reactive({
todos: ['learn Vue', 'build app']
})
state.todos.push('deploy app')
```
2. toRefs
toRefs是一个将响应式对象转换成普通对象的API。它将对象的所有属性都转换成ref对象,这样可以方便地在模板中使用。
```javascript
import { reactive, toRefs } from 'vue'
const state = reactive({
todos: ['learn Vue', 'build app']
})
const refs = toRefs(state)
console.log(refs.todos.value) // ['learn Vue', 'build app']
```
3. computed
computed是Vue3中用来创建计算属性的API。它可以接收一个getter函数和一个可选的setter函数。getter函数返回一个计算结果,setter函数用来更新计算结果。
```javascript
import { reactive, computed } from 'vue'
const state = reactive({
todos: ['learn Vue', 'build app']
})
const todoCount = computed(() => {
return state.todos.length
})
console.log(todoCount.value) // 2
```
4. watchEffect
watchEffect是一个API,它可以让你监听响应式数据的变化,并在变化时执行一个函数。它类似于Vue2中的watch,但更加简洁。
```javascript
import { reactive, watchEffect } from 'vue'
const state = reactive({
todos: ['learn Vue', 'build app']
})
watchEffect(() => {
console.log('todos changed', state.todos)
})
```
以上是Vue3中操作数组的一些API和语法糖,它们可以让你更加方便地创建响应式的数组,并在模板中使用。
阅读全文
相关推荐
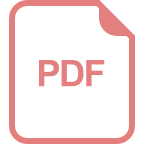
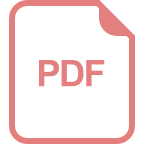
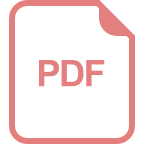















