CANoe 通过USBTMC控制程控电源代码
时间: 2024-03-02 11:29:03 浏览: 205
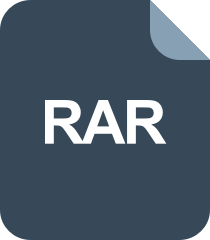
usb控制器源代码

下面是一个使用CANoe通过USBTMC控制程控电源的示例代码:
```
#include <windows.h>
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#include <tchar.h>
#include <visa.h>
ViSession defaultRM;
ViSession instr;
void main()
{
ViStatus status;
ViUInt32 retCnt;
char instrDescriptor[VI_FIND_BUFLEN];
ViFindList findList;
ViUInt32 numInstrs;
ViChar instrModel[128];
ViChar instrSerial[128];
ViChar instrFirmware[128];
ViChar instrOptions[128];
ViBoolean idQuery;
ViBoolean resetInstr;
ViUInt32 timeout = 2000;
char cmd[256];
char resp[256];
// Initialize VISA
status = viOpenDefaultRM(&defaultRM);
if (status < VI_SUCCESS) {
printf("Could not open a session to the VISA Resource Manager!\n");
return;
}
// Find the instrument
status = viFindRsrc(defaultRM, "USB[0-9]*::0xXXXX::0xXXXX::XXXXXXXX::INSTR", &findList, &numInstrs, instrDescriptor);
if (status < VI_SUCCESS) {
printf("Could not find the instrument!\n");
viClose(defaultRM);
return;
}
// Open the instrument session
status = viOpen(defaultRM, instrDescriptor, VI_NULL, VI_NULL, &instr);
if (status < VI_SUCCESS) {
printf("Could not open a session to the instrument!\n");
viClose(defaultRM);
return;
}
// Initialize the instrument
idQuery = VI_TRUE;
resetInstr = VI_TRUE;
status = viSetAttribute(instr, VI_ATTR_TMO_VALUE, timeout);
if (status < VI_SUCCESS) {
printf("Could not set timeout attribute!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viSetAttribute(instr, VI_ATTR_TERMCHAR_EN, VI_FALSE);
if (status < VI_SUCCESS) {
printf("Could not set termchar attribute!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viSetAttribute(instr, VI_ATTR_SEND_END_EN, VI_TRUE);
if (status < VI_SUCCESS) {
printf("Could not set send end attribute!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viSetAttribute(instr, VI_ATTR_TERMCHAR, '\n');
if (status < VI_SUCCESS) {
printf("Could not set termchar attribute!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viSetAttribute(instr, VI_ATTR_TERMCHAR_EN, VI_TRUE);
if (status < VI_SUCCESS) {
printf("Could not set termchar attribute!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viSetAttribute(instr, VI_ATTR_TMO_VALUE, timeout);
if (status < VI_SUCCESS) {
printf("Could not set timeout attribute!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viGetAttribute(instr, VI_ATTR_MODEL_NAME, instrModel);
if (status < VI_SUCCESS) {
printf("Could not get instrument model name!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viGetAttribute(instr, VI_ATTR_SERIAL_NUM, instrSerial);
if (status < VI_SUCCESS) {
printf("Could not get instrument serial number!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viGetAttribute(instr, VI_ATTR_SW_VERSION, instrFirmware);
if (status < VI_SUCCESS) {
printf("Could not get instrument firmware version!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viGetAttribute(instr, VI_ATTR_OPTIONS, instrOptions);
if (status < VI_SUCCESS) {
printf("Could not get instrument options!\n");
viClose(instr);
viClose(defaultRM);
return;
}
printf("Instrument found:\n");
printf("Model: %s\n", instrModel);
printf("Serial: %s\n", instrSerial);
printf("Firmware: %s\n", instrFirmware);
printf("Options: %s\n", instrOptions);
// Send commands to the instrument
sprintf(cmd, "OUTPut ON");
status = viWrite(instr, (ViBuf)cmd, (ViUInt32)strlen(cmd), &retCnt);
if (status < VI_SUCCESS) {
printf("Could not send command to the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
sprintf(cmd, "VOLTage 5.0");
status = viWrite(instr, (ViBuf)cmd, (ViUInt32)strlen(cmd), &retCnt);
if (status < VI_SUCCESS) {
printf("Could not send command to the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
sprintf(cmd, "CURRent 1.0");
status = viWrite(instr, (ViBuf)cmd, (ViUInt32)strlen(cmd), &retCnt);
if (status < VI_SUCCESS) {
printf("Could not send command to the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
// Query the instrument
sprintf(cmd, "MEASure:VOLTage?");
status = viWrite(instr, (ViBuf)cmd, (ViUInt32)strlen(cmd), &retCnt);
if (status < VI_SUCCESS) {
printf("Could not send command to the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viRead(instr, (ViBuf)resp, 256, &retCnt);
if (status < VI_SUCCESS) {
printf("Could not read response from the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
printf("Voltage: %s\n", resp);
sprintf(cmd, "MEASure:CURRent?");
status = viWrite(instr, (ViBuf)cmd, (ViUInt32)strlen(cmd), &retCnt);
if (status < VI_SUCCESS) {
printf("Could not send command to the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
status = viRead(instr, (ViBuf)resp, 256, &retCnt);
if (status < VI_SUCCESS) {
printf("Could not read response from the instrument!\n");
viClose(instr);
viClose(defaultRM);
return;
}
printf("Current: %s\n", resp);
// Close the instrument session
viClose(instr);
// Close the default resource manager session
viClose(defaultRM);
}
```
在代码中,我们使用了VISA库(Virtual Instrument Software Architecture),通过USB连接程控电源,控制电源的输出电压和电流,并查询电源的输出电压和电流。你需要将代码中的"USB[0-9]*::0xXXXX::0xXXXX::XXXXXXXX::INSTR"替换成你的程控电源的VISA资源描述符。
阅读全文
相关推荐
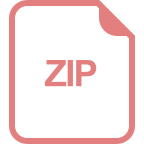



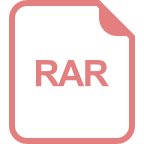
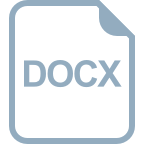
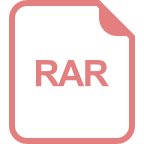
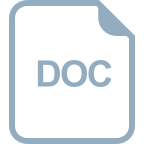







