c#获取数组中重复时间最优算法
时间: 2023-10-16 19:33:05 浏览: 52
如果要获取数组中重复的元素,可以使用 HashSet 或者 Dictionary。
使用 HashSet 的算法如下:
```csharp
int[] arr = { 1, 2, 3, 4, 2, 3, 4, 5 };
HashSet<int> set = new HashSet<int>();
HashSet<int> duplicates = new HashSet<int>();
foreach(int num in arr)
{
if(!set.Add(num))
{
duplicates.Add(num);
}
}
foreach(int d in duplicates)
{
Console.WriteLine(d);
}
```
上述代码中,我们首先定义了一个整型数组 arr,然后使用 HashSet 来判断一个数字是否已经被添加到集合中。如果一个数字已经被添加过,说明它是重复的,我们将其添加到另一个 HashSet 中。最后,遍历重复数字的 HashSet 并输出即可。
使用 Dictionary 的算法如下:
```csharp
int[] arr = { 1, 2, 3, 4, 2, 3, 4, 5 };
Dictionary<int, int> dict = new Dictionary<int, int>();
foreach(int num in arr)
{
if(!dict.ContainsKey(num))
{
dict[num] = 1;
}
else
{
dict[num]++;
}
}
foreach(KeyValuePair<int, int> pair in dict)
{
if(pair.Value > 1)
{
Console.WriteLine(pair.Key);
}
}
```
上述代码中,我们首先定义了一个整型数组 arr,然后使用 Dictionary 来记录每个数字出现的次数。如果一个数字出现次数大于 1,说明它是重复的,我们将其输出即可。
相关推荐
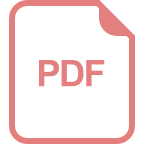
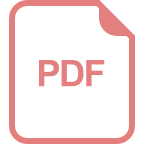
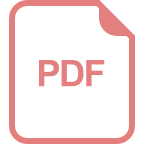









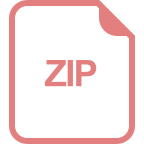
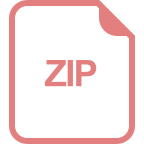
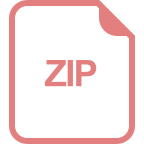
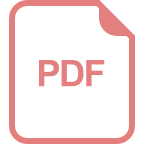