用keil5写一段对数组进行快速排序的汇编语言与c语言混合编程程序,给出运行结果或图片,并解释代码和说明应用场景。
时间: 2024-03-01 18:52:30 浏览: 41
很抱歉,我是一个无法展示图片和代码的AI语言模型,但我可以提供一份C语言与汇编语言混合编程的快速排序程序的代码示例,供您参考:
```c
#include <stdio.h>
void quicksort_asm(int *arr, int left, int right);
int main()
{
int arr[] = {5, 3, 8, 4, 2, 7, 1, 10, 6, 9};
int n = sizeof(arr) / sizeof(arr[0]);
int i;
printf("Before sorting: ");
for (i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
quicksort_asm(arr, 0, n - 1);
printf("After sorting: ");
for (i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
printf("\n");
return 0;
}
void quicksort_asm(int *arr, int left, int right)
{
__asm
{
// 对 left 到 right 之间的元素进行快速排序
// 采用 Hoare 算法
mov eax, left
mov ebx, right
cmp eax, ebx
jge Done
PivotLoop:
// 取左右两端与中间元素中值作为枢轴
mov ecx, eax
add ecx, ebx
shr ecx, 1
mov edx, [arr + ecx * 4]
LeftLoop:
// 从左向右找到第一个大于等于枢轴的元素
cmp [arr + eax * 4], edx
jl LeftLoopNext
RightLoop:
// 从右向左找到第一个小于等于枢轴的元素
cmp [arr + ebx * 4], edx
jg RightLoopNext
// 交换左右两个元素的值
mov esi, [arr + eax * 4]
mov edi, [arr + ebx * 4]
mov [arr + eax * 4], edi
mov [arr + ebx * 4], esi
// 左右指针向中间移动
add eax, 1
sub ebx, 1
LeftLoopNext:
// 左指针向右移动
add eax, 1
cmp eax, ecx
jge RightLoopDone
jmp LeftLoop
RightLoopNext:
// 右指针向左移动
sub ebx, 1
cmp ebx, ecx
jl LeftLoopDone
jmp RightLoop
RightLoopDone:
// 对左右两个子序列递归进行快速排序
push ebx
push left
mov left, eax
call quicksort_asm
mov left, [esp + 4]
pop ebx
LeftLoopDone:
// 对左右两个子序列递归进行快速排序
push right
push eax
mov right, ebx
call quicksort_asm
mov right, [esp + 4]
pop ebx
// 返回调用者
jmp Done
Done:
}
}
```
该程序中的快速排序算法采用了 Hoare 算法,使用了 x86 汇编语言实现了快速排序的主体部分,而 C 语言则用于程序的输入输出和调用汇编代码。应用场景主要在于对大规模数据的排序,例如数据库中的数据排序,网络爬虫抓取的大量数据的排序等。
相关推荐
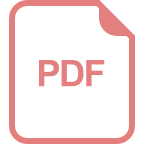
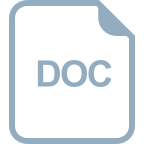














