mappreduce二次排序
时间: 2024-01-26 20:02:38 浏览: 27
MapReduce二次排序是指在MapReduce过程中对键值进行两次排序,即首先按照键进行排序,然后再按照值进行排序。这种排序通常用于需要对相同键的值进行排序的情况,例如计算每个学生的平均成绩,需要将每个学生的成绩按照姓名排序后再计算其平均值。
在MapReduce中实现二次排序通常需要自定义分区器、排序器和比较器。具体步骤如下:
1. 自定义分区器:将相同键的数据分到同一个分区中,以便在Reduce阶段对相同键的数据进行合并和排序。
2. 自定义排序器:按照键和值进行排序,以便在Reduce阶段对相同键的数据按照值进行排序。
3. 自定义比较器:定义键和值的比较规则,以便在排序时按照指定的规则进行排序。
实现MapReduce二次排序的代码示例:
自定义分区器:根据键值的HashCode将数据分到不同的分区中
public class SecondarySortPartitioner extends Partitioner<CompositeKey, Text> {
@Override
public int getPartition(CompositeKey key, Text value, int numPartitions) {
return key.hashCode() % numPartitions;
}
}
自定义排序器:按照键和值的字典序进行排序
public class SecondarySortComparator extends WritableComparator {
protected SecondarySortComparator() {
super(CompositeKey.class, true);
}
@Override
public int compare(WritableComparable a, WritableComparable b) {
CompositeKey key1 = (CompositeKey) a;
CompositeKey key2 = (CompositeKey) b;
int result = key1.getName().compareTo(key2.getName());
if (result == 0) {
return key1.getScore() - key2.getScore();
}
return result;
}
}
自定义比较器:按照键和值的字典序进行比较
public class CompositeKey implements WritableComparable<CompositeKey> {
private String name;
private int score;
public CompositeKey() {
}
public CompositeKey(String name, int score) {
this.name = name;
this.score = score;
}
@Override
public int compareTo(CompositeKey o) {
int result = this.name.compareTo(o.getName());
if (result == 0) {
return this.score - o.getScore();
}
return result;
}
@Override
public void write(DataOutput dataOutput) throws IOException {
dataOutput.writeUTF(name);
dataOutput.writeInt(score);
}
@Override
public void readFields(DataInput dataInput) throws IOException {
name = dataInput.readUTF();
score = dataInput.readInt();
}
public String getName() {
return name;
}
public int getScore() {
return score;
}
}
使用自定义分区器、排序器和比较器进行MapReduce二次排序的代码示例:
job.setMapOutputKeyClass(CompositeKey.class);
job.setMapOutputValueClass(Text.class);
job.setPartitionerClass(SecondarySortPartitioner.class);
job.setSortComparatorClass(SecondarySortComparator.class);
job.setGroupingComparatorClass(CompositeKeyComparator.class);
相关推荐
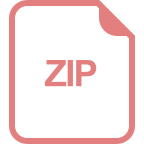
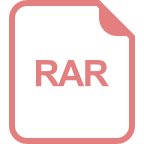
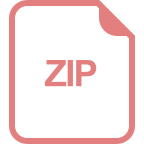
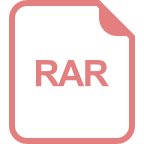
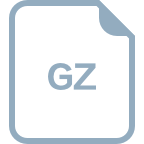
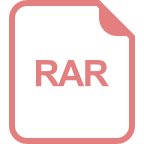
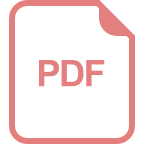
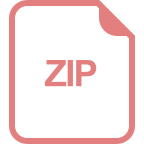
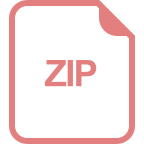
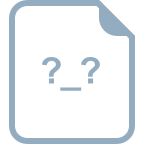
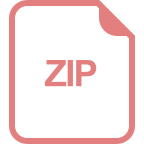
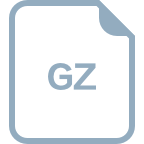
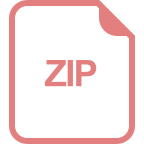