JavaScript写后端连接数据库mysql并渲染到前端网页中,有格式要求
时间: 2024-03-20 21:42:18 浏览: 20
好的,下面是一个使用 Node.js 和 MySQL 连接数据库,并将数据渲染到前端网页中的示例代码。
首先,需要安装 `mysql` 和 `express` 模块。
```bash
npm install mysql express
```
然后,创建一个 `app.js` 文件,编写以下代码:
```javascript
const express = require('express');
const mysql = require('mysql');
// 创建 MySQL 连接池
const pool = mysql.createPool({
host: 'localhost',
user: 'username',
password: 'password',
database: 'mydb',
});
// 创建 Express 应用程序
const app = express();
// 处理根路径的 GET 请求
app.get('/', (req, res) => {
// 从数据库中获取数据
pool.query('SELECT * FROM users', (error, results, fields) => {
if (error) throw error;
// 将数据渲染到 HTML 页面中
const html = `
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Users</title>
</head>
<body>
<h1>Users</h1>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
${results.map(user => `
<tr>
<td>${user.id}</td>
<td>${user.name}</td>
<td>${user.email}</td>
</tr>
`).join('')}
</tbody>
</table>
</body>
</html>
`;
// 发送 HTML 页面到客户端
res.send(html);
});
});
// 启动服务器
app.listen(3000, () => {
console.log('Server is running at http://localhost:3000');
});
```
代码说明:
1. 首先,使用 `mysql` 模块创建一个 MySQL 连接池。
2. 然后,使用 `express` 模块创建一个 Express 应用程序。
3. 处理根路径的 GET 请求,从数据库中获取数据,并将数据渲染到 HTML 页面中。
4. 启动服务器,监听 3000 端口。
注意:以上代码只是一个简单示例,具体实现要根据具体的需求进行修改。
相关推荐
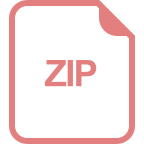
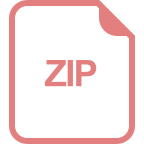
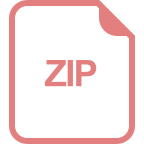














