帮我生成dht11的驱动代码
时间: 2023-03-24 11:04:29 浏览: 92
你好,我可以为您生成 DHT11 的驱动代码。以下是示例代码:
#include <dht11.h>
dht11 DHT11;
void setup() {
Serial.begin(960);
}
void loop() {
int chk = DHT11.read(7); // 读取 DHT11 传感器数据,引脚为 7
Serial.print("Humidity (%): ");
Serial.println(DHT11.humidity);
Serial.print("Temperature (°C): ");
Serial.println(DHT11.temperature);
delay(200); // 2 秒后再次读取数据
}
希望这可以帮助到您。
相关问题
生成驱动DHT11的C语言代码
以下是一个基于STM32F4的C语言代码示例,用于驱动DHT11传感器:
```c
#include "stm32f4xx.h"
#include "dht11.h"
void delay_us(uint32_t us)
{
uint32_t count = us * (SystemCoreClock / 1000000) / 3;
while (count--);
}
void DHT11_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_UP;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_SetBits(GPIOA, GPIO_Pin_0);
delay_us(1000);
}
uint8_t DHT11_Read_Byte(void)
{
uint8_t i, j, byte = 0;
for (j = 0; j < 8; j++)
{
while (!GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0));
delay_us(40);
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0) == 0)
{
byte &= ~(1 << (7 - j));
}
else
{
byte |= (1 << (7 - j));
while (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0));
}
}
return byte;
}
DHT11_Data_TypeDef DHT11_Read_Data(void)
{
DHT11_Data_TypeDef dht11_data;
uint8_t i, checksum;
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
delay_us(18000);
GPIO_SetBits(GPIOA, GPIO_Pin_0);
delay_us(30);
GPIO_ResetBits(GPIOA, GPIO_Pin_0);
delay_us(40);
GPIO_SetBits(GPIOA, GPIO_Pin_0);
while (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0));
while (!GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0));
while (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_0));
dht11_data.humidity = DHT11_Read_Byte();
dht11_data.temperature = DHT11_Read_Byte();
checksum = DHT11_Read_Byte();
GPIO_SetBits(GPIOA, GPIO_Pin_0);
if (checksum == (dht11_data.temperature + dht11_data.humidity))
{
return dht11_data;
}
else
{
dht11_data.humidity = 0;
dht11_data.temperature = 0;
return dht11_data;
}
}
```
该代码使用了STM32F4的GPIO口控制DHT11传感器,并使用delay_us函数等待DHT11的响应。在读取温湿度数据时,先发送起始信号,然后通过读取DHT11传感器的响应数据,计算出温度和湿度,并验证校验和。最后,返回温湿度数据结构体。
生成stm32的DHT11代码
以下是基于STM32 HAL库的DHT11驱动代码示例:
```c
#include "stm32f4xx_hal.h"
#define DHT11_PORT GPIOA
#define DHT11_PIN GPIO_PIN_0
void delay_us(uint32_t us)
{
uint32_t count = us * (SystemCoreClock / 1000000) / 5;
while(count--);
}
void DHT11_Start(void)
{
HAL_GPIO_WritePin(DHT11_PORT, DHT11_PIN, GPIO_PIN_RESET);
delay_us(18000);
HAL_GPIO_WritePin(DHT11_PORT, DHT11_PIN, GPIO_PIN_SET);
delay_us(20);
HAL_GPIO_WritePin(DHT11_PORT, DHT11_PIN, GPIO_PIN_RESET);
}
uint8_t DHT11_Check(void)
{
uint8_t response = 1;
uint16_t count = 0;
while(HAL_GPIO_ReadPin(DHT11_PORT, DHT11_PIN) == GPIO_PIN_SET)
{
count++;
if(count > 10000)
{
response = 0;
break;
}
}
return response;
}
uint8_t DHT11_Read_Bit(void)
{
uint8_t response = 1;
uint16_t count = 0;
while(HAL_GPIO_ReadPin(DHT11_PORT, DHT11_PIN) == GPIO_PIN_RESET)
{
count++;
if(count > 10000)
{
response = 0;
break;
}
}
delay_us(40);
if(HAL_GPIO_ReadPin(DHT11_PORT, DHT11_PIN) == GPIO_PIN_SET)
{
response = 1;
}
else
{
response = 0;
}
count = 0;
while(HAL_GPIO_ReadPin(DHT11_PORT, DHT11_PIN) == GPIO_PIN_SET)
{
count++;
if(count > 10000)
{
response = 0;
break;
}
}
return response;
}
uint8_t DHT11_Read_Byte(void)
{
uint8_t i, temp = 0;
for(i = 0; i < 8; i++)
{
temp |= (DHT11_Read_Bit() << (7 - i));
}
return temp;
}
uint8_t DHT11_Read_Data(uint8_t *humidity, uint8_t *temperature)
{
uint8_t response = 0;
uint8_t buffer[5];
DHT11_Start();
if(DHT11_Check() == 0)
{
response = 1;
buffer[0] = DHT11_Read_Byte();
buffer[1] = DHT11_Read_Byte();
buffer[2] = DHT11_Read_Byte();
buffer[3] = DHT11_Read_Byte();
buffer[4] = DHT11_Read_Byte();
if(buffer[4] == (buffer[0] + buffer[1] + buffer[2] + buffer[3]))
{
*humidity = buffer[0];
*temperature = buffer[2];
}
else
{
response = 2;
}
}
return response;
}
```
使用方法:
1. 在`main.c`文件中添加上述代码。
2. 在`main`函数中调用`DHT11_Read_Data`函数,读取温湿度数据。
示例代码:
```c
#include "stm32f4xx_hal.h"
uint8_t humidity, temperature;
int main(void)
{
HAL_Init();
/* 初始化GPIO */
GPIO_InitTypeDef GPIO_InitStruct;
__HAL_RCC_GPIOA_CLK_ENABLE();
GPIO_InitStruct.Pin = DHT11_PIN;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH;
HAL_GPIO_Init(DHT11_PORT, &GPIO_InitStruct);
HAL_GPIO_WritePin(DHT11_PORT, DHT11_PIN, GPIO_PIN_RESET);
/* 读取温湿度数据 */
uint8_t response = DHT11_Read_Data(&humidity, &temperature);
if(response == 0)
{
/* 温湿度数据读取成功 */
printf("湿度:%d%%,温度:%d℃\n", humidity, temperature);
}
else if(response == 1)
{
/* DHT11无响应 */
printf("DHT11无响应\n");
}
else if(response == 2)
{
/* 校验和错误 */
printf("校验和错误\n");
}
while(1);
}
```
阅读全文
相关推荐
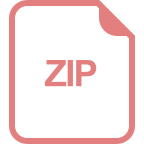
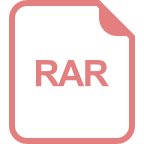

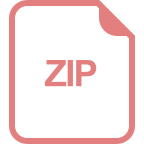
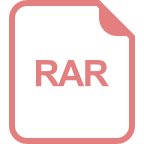
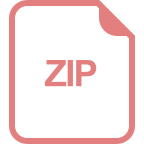
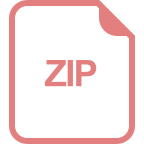
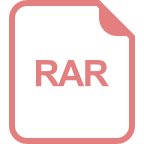
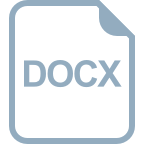
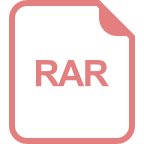
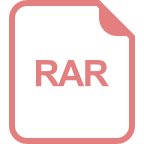
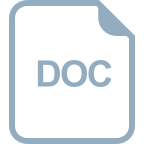
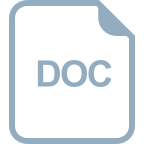
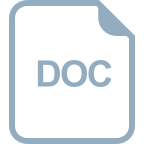
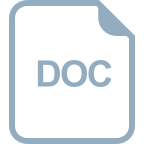