代码生成理财产品信息管理系统
时间: 2023-10-30 17:04:54 浏览: 42
以下是一个简单的代码生成理财产品信息管理系统的代码示例:
```python
# 导入必要的模块
import csv
# 定义理财产品类
class FinancialProduct:
def __init__(self, product_id, name, rate, duration):
self.product_id = product_id
self.name = name
self.rate = rate
self.duration = duration
def __str__(self):
return f"{self.product_id}, {self.name}, {self.rate}, {self.duration}"
# 定义理财产品信息管理系统类
class FinancialProductManagementSystem:
def __init__(self, file_path):
self.file_path = file_path
self.products = []
# 从 CSV 文件中加载理财产品信息
def load_products(self):
with open(self.file_path, "r") as file:
reader = csv.reader(file)
for row in reader:
product = FinancialProduct(row[0], row[1], float(row[2]), int(row[3]))
self.products.append(product)
# 添加新的理财产品
def add_product(self, product):
self.products.append(product)
# 保存理财产品信息到 CSV 文件中
def save_products(self):
with open(self.file_path, "w", newline="") as file:
writer = csv.writer(file)
for product in self.products:
writer.writerow([product.product_id, product.name, product.rate, product.duration])
# 根据理财产品名称查找产品
def find_product_by_name(self, name):
for product in self.products:
if product.name == name:
return product
return None
# 根据理财产品 ID 删除产品
def delete_product_by_id(self, product_id):
for product in self.products:
if product.product_id == product_id:
self.products.remove(product)
break
# 使用示例
# 创建理财产品信息管理系统对象
system = FinancialProductManagementSystem("products.csv")
# 加载理财产品信息
system.load_products()
# 添加新的理财产品
product = FinancialProduct("P003", "高级定期理财", 4.5, 365)
system.add_product(product)
# 保存理财产品信息到 CSV 文件中
system.save_products()
# 查找理财产品
product = system.find_product_by_name("高级定期理财")
print(product)
# 删除理财产品
system.delete_product_by_id("P003")
# 保存理财产品信息到 CSV 文件中
system.save_products()
```
该代码示例中定义了两个类,一个是 `FinancialProduct` 类用于表示理财产品,另一个是 `FinancialProductManagementSystem` 类用于管理理财产品信息。其中,`FinancialProductManagementSystem` 类提供了一些方法用于加载、保存、添加、删除和查找理财产品信息,并且使用 CSV 文件存储数据。你可以根据需求调整代码,以实现更复杂的功能。
相关推荐
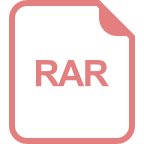
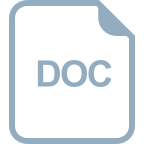
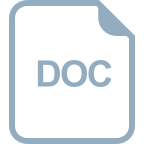














