QT 超大字符串写入文件进度
时间: 2023-07-25 15:19:54 浏览: 105
如果你需要在QT中将超大字符串写入文件,并且需要显示写入的进度,可以使用`QFile`和`QTextStream`对象协作完成。具体来说,你可以使用`QTextStream`对象格式化超大字符串,然后将其从`QTextStream`对象中读取出来,并将其写入到一个文件中,并在每次写入一定数量的字符后,更新进度条的值。
以下是一个示例代码:
```cpp
#include <QMainWindow>
#include <QProgressBar>
#include <QPushButton>
#include <QHBoxLayout>
#include <QFile>
#include <QTextStream>
#include <QString>
class MainWindow : public QMainWindow {
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr): QMainWindow(parent) {
QPushButton *button = new QPushButton("Write to File");
connect(button, &QPushButton::clicked, this, &MainWindow::writeToFile);
progressBar = new QProgressBar();
QHBoxLayout *layout = new QHBoxLayout();
layout->addWidget(button);
layout->addWidget(progressBar);
QWidget *centralWidget = new QWidget();
centralWidget->setLayout(layout);
setCentralWidget(centralWidget);
}
private slots:
void writeToFile() {
QString format = "This is a formatted string with arguments: %1, %2, %3\n";
QString arg1 = "argument 1";
QString arg2 = "argument 2";
QString arg3 = "argument 3";
QFile file("output.txt");
if (!file.open(QIODevice::WriteOnly | QIODevice::Text))
return;
QTextStream out(&file);
out.setCodec("UTF-8");
int totalLength = format.length() + arg1.length() + arg2.length() + arg3.length();
int writtenLength = 0;
while (writtenLength < totalLength) {
QString result = format.arg(arg1).arg(arg2).arg(arg3);
int length = result.length();
out << result;
writtenLength += length;
progressBar->setValue((writtenLength * 100) / totalLength);
arg1 += arg1;
arg2 += arg2;
arg3 += arg3;
}
file.close();
}
private:
QProgressBar *progressBar;
};
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
```
在上面的示例中,我们创建了一个显示进度条和一个"Write to File"按钮的主窗口。当点击按钮时,会将超大字符串格式化后写入到文件中,并根据写入的进度更新进度条的值。在`writeToFile`函数中,我们首先打开文件,然后使用`QTextStream`对象`out`将超大字符串写入到文件中。在每次写入一定数量的字符后,我们更新进度条的值,并将超大字符串中的参数进行指数级增长,以模拟超大字符串的情况。
需要注意的是,在这个示例中,我们使用`UTF-8`编码将数据写入到文件中。如果你需要使用其他编码,请根据需要修改对应的编码名称。
阅读全文
相关推荐
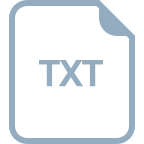
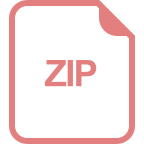
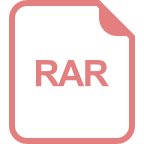
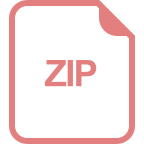
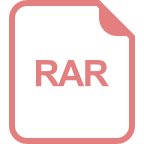
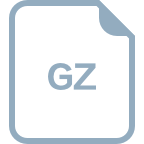
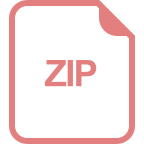
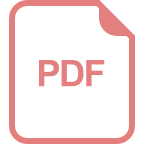
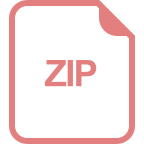
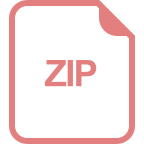
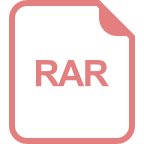
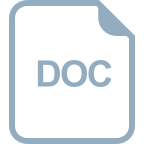
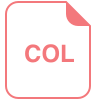
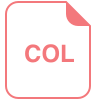
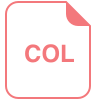

