用java写编写一个程序,实现复数运算,要求如下: 1) 设计并实现复数类。 2) 设计并实现复数的加、减、乘、除运算。以提供任意数值类型数据与复数对象实例自身的运算功能。
时间: 2024-03-06 21:52:21 浏览: 87
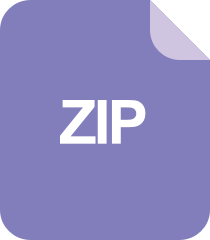
spring 异步编程样例
好的,我可以帮你解答这个问题。
首先,我们需要定义一个复数类,它有两个属性:实部和虚部。代码如下:
```
public class Complex {
private double real; // 实部
private double imag; // 虚部
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// getter 和 setter 方法省略
// 加法运算
public Complex add(Complex other) {
double newReal = this.real + other.real;
double newImag = this.imag + other.imag;
return new Complex(newReal, newImag);
}
// 减法运算
public Complex subtract(Complex other) {
double newReal = this.real - other.real;
double newImag = this.imag - other.imag;
return new Complex(newReal, newImag);
}
// 乘法运算
public Complex multiply(Complex other) {
double newReal = this.real * other.real - this.imag * other.imag;
double newImag = this.real * other.imag + this.imag * other.real;
return new Complex(newReal, newImag);
}
// 除法运算
public Complex divide(Complex other) {
double denominator = other.real * other.real + other.imag * other.imag;
double newReal = (this.real * other.real + this.imag * other.imag) / denominator;
double newImag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(newReal, newImag);
}
@Override
public String toString() {
return this.real + " + " + this.imag + "i";
}
}
```
在这个类中,我们定义了四种运算方法:加法、减法、乘法、除法。这些方法都返回一个新的复数对象,而不是修改原来的对象。
接下来,我们可以在主函数中测试这个复数类的运算方法。例如:
```
public static void main(String[] args) {
Complex c1 = new Complex(1, 2);
Complex c2 = new Complex(3, 4);
System.out.println(c1.add(c2)); // 输出 4.0 + 6.0i
System.out.println(c1.subtract(c2)); // 输出 -2.0 - 2.0i
System.out.println(c1.multiply(c2)); // 输出 -5.0 + 10.0i
System.out.println(c1.divide(c2)); // 输出 0.44 + 0.08i
}
```
以上就是用 Java 实现复数运算的方法。注意,这里的复数类只是一个简单的实现,如果需要更高级的功能(例如求模长、求辐角等),可以自行扩展。
阅读全文
相关推荐
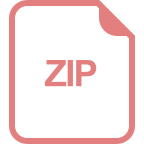
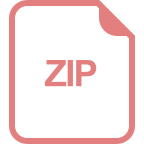
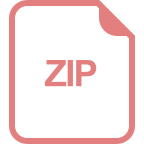
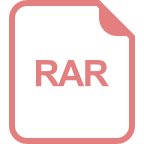
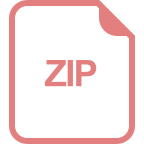
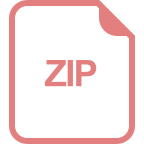
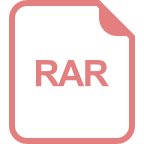
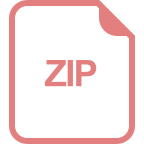
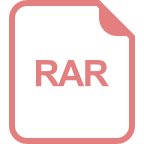
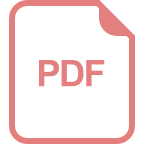
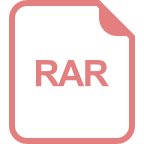