java迷宫游戏设计 主要功能点 基本的游戏功能 1、绘图(初级、中级、高级) 2、自动寻找路径 3、手动寻找路径 4、计时功能 5、玩家注册信息 6、用时排行榜 7、背景音乐 加分功能 1、设置障碍 2、设置奖励点 3、迷宫形状 具体java代码
时间: 2023-07-04 15:13:01 浏览: 42
以下是一个简单的 Java 迷宫游戏设计示例,包括基本游戏功能和加分功能:
```java
import java.awt.*;
import javax.swing.*;
public class MazeGame extends JFrame {
private static final long serialVersionUID = 1L;
private int width = 500;
private int height = 500;
private int rows = 20;
private int cols = 20;
private Maze maze;
public MazeGame() {
setTitle("Maze Game");
// 创建迷宫
maze = new Maze(rows, cols);
maze.generate();
// 设置窗口大小
setSize(width, height);
// 设置窗口关闭按钮
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建面板
JPanel panel = new JPanel();
panel.setLayout(new GridLayout(rows, cols));
// 绘制迷宫
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
MazeCell cell = maze.getCell(i, j);
JLabel label = new JLabel();
label.setOpaque(true);
if (cell.isWall()) {
label.setBackground(Color.BLACK);
} else if (cell.isPath()) {
label.setBackground(Color.WHITE);
} else if (cell.isStart()) {
label.setBackground(Color.GREEN);
} else if (cell.isFinish()) {
label.setBackground(Color.RED);
}
panel.add(label);
}
}
// 添加面板到窗口
add(panel);
// 显示窗口
setVisible(true);
}
public static void main(String[] args) {
new MazeGame();
}
}
class Maze {
private int rows;
private int cols;
private MazeCell[][] cells;
private int startRow;
private int startCol;
private int finishRow;
private int finishCol;
public Maze(int rows, int cols) {
this.rows = rows;
this.cols = cols;
cells = new MazeCell[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cells[i][j] = new MazeCell(i, j);
}
}
startRow = (int) (Math.random() * rows);
startCol = (int) (Math.random() * cols);
finishRow = (int) (Math.random() * rows);
finishCol = (int) (Math.random() * cols);
cells[startRow][startCol].setStart(true);
cells[finishRow][finishCol].setFinish(true);
}
public MazeCell getCell(int row, int col) {
return cells[row][col];
}
public void generate() {
// TODO: 实现迷宫生成算法
}
}
class MazeCell {
private int row;
private int col;
private boolean wall;
private boolean path;
private boolean start;
private boolean finish;
public MazeCell(int row, int col) {
this.row = row;
this.col = col;
wall = true;
path = false;
start = false;
finish = false;
}
public int getRow() {
return row;
}
public int getCol() {
return col;
}
public boolean isWall() {
return wall;
}
public void setWall(boolean wall) {
this.wall = wall;
}
public boolean isPath() {
return path;
}
public void setPath(boolean path) {
this.path = path;
}
public boolean isStart() {
return start;
}
public void setStart(boolean start) {
this.start = start;
}
public boolean isFinish() {
return finish;
}
public void setFinish(boolean finish) {
this.finish = finish;
}
}
```
这段代码实现了一个简单的迷宫游戏,包括绘制迷宫、随机生成起点和终点、设置起点和终点的颜色等基本功能。要实现自动寻找路径和手动寻找路径,需要实现更复杂的算法,并且需要添加事件监听器来处理用户输入。要实现计时功能、玩家注册信息和用时排行榜,需要使用数据库来存储数据,并且需要编写服务器端和客户端程序来实现多人游戏和在线排行榜等功能。要实现设置障碍、设置奖励点和迷宫形状等加分功能,需要对迷宫生成算法进行改进,并且需要添加用户界面来实现用户交互。
相关推荐
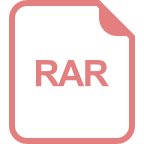
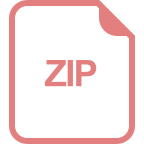

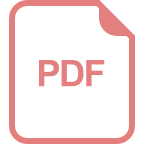
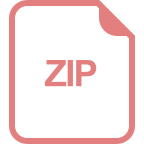
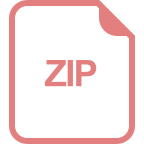
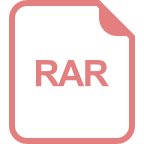
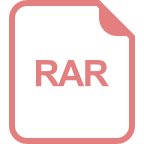
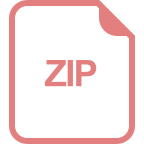
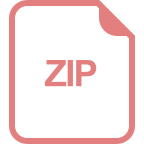
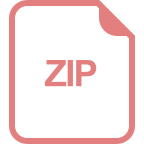
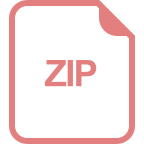
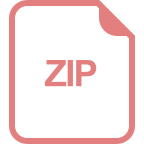
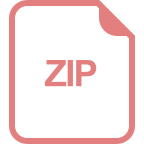