python实现以下有界面的程序功能,并用类封装,加上main函数:按alt键自动截图,并将所有截图组成截图集合,可以随时保存截图集合为文件或新建截图集合文件,或打开截图集合文件,通过打开或新建截图集合文件后按左右方向键可以依次浏览截图集合中的截图。
时间: 2024-04-30 12:19:32 浏览: 59
以下是一个简单的实现方案:
```python
import tkinter as tk
import os
import time
import shutil
import keyboard
from PIL import ImageGrab
class ScreenshotApp:
def __init__(self, master):
self.master = master
self.master.title("Screenshot App")
# 创建界面组件
self.btn_new = tk.Button(self.master, text="New Collection", command=self.new_collection)
self.btn_save = tk.Button(self.master, text="Save Collection", command=self.save_collection)
self.btn_open = tk.Button(self.master, text="Open Collection", command=self.open_collection)
self.btn_prev = tk.Button(self.master, text="Previous", command=self.prev_image)
self.btn_next = tk.Button(self.master, text="Next", command=self.next_image)
self.lbl_image = tk.Label(self.master)
# 布局界面组件
self.btn_new.grid(row=0, column=0)
self.btn_save.grid(row=0, column=1)
self.btn_open.grid(row=0, column=2)
self.btn_prev.grid(row=1, column=0, sticky="w")
self.btn_next.grid(row=1, column=2, sticky="e")
self.lbl_image.grid(row=2, column=0, columnspan=3)
# 初始化截图集合
self.collection = []
self.current_index = -1
# 监听键盘事件
keyboard.add_hotkey("alt", self.capture_screenshot)
def new_collection(self):
# 创建新的截图集合
self.collection = []
self.current_index = -1
self.show_image()
def save_collection(self):
# 保存截图集合为文件
filename = tk.filedialog.asksaveasfilename(defaultextension=".txt")
if filename:
with open(filename, "w") as f:
for image_path in self.collection:
f.write(image_path + "\n")
def open_collection(self):
# 打开截图集合文件
filename = tk.filedialog.askopenfilename(defaultextension=".txt")
if filename:
with open(filename, "r") as f:
self.collection = [line.strip() for line in f.readlines()]
self.current_index = 0
self.show_image()
def capture_screenshot(self):
# 截图并添加到集合中
image = ImageGrab.grab()
timestamp = int(time.time())
image_path = f"{timestamp}.png"
image.save(image_path)
self.collection.append(image_path)
self.current_index = len(self.collection) - 1
self.show_image()
def prev_image(self):
# 显示上一张图片
if self.current_index > 0:
self.current_index -= 1
self.show_image()
def next_image(self):
# 显示下一张图片
if self.current_index < len(self.collection) - 1:
self.current_index += 1
self.show_image()
def show_image(self):
# 显示当前图片
if self.collection:
image_path = self.collection[self.current_index]
image = tk.PhotoImage(file=image_path)
self.lbl_image.configure(image=image)
self.lbl_image.image = image
def main():
root = tk.Tk()
app = ScreenshotApp(root)
root.mainloop()
if __name__ == "__main__":
main()
```
这个程序使用了 tkinter 和 keyboard 库来创建界面和处理键盘事件,使用了 PIL 库来截图和显示图片。在程序运行时,按下 Alt 键即可截图并添加到截图集合中,通过界面上的按钮可以新建、保存、打开截图集合文件,通过左右方向键可以浏览截图集合中的截图。截图集合默认保存在程序运行的当前目录下。
阅读全文
相关推荐
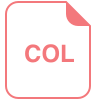
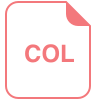
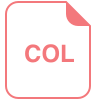
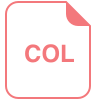
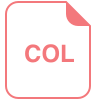
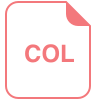
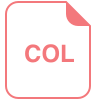
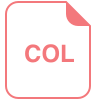
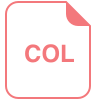
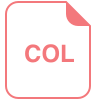
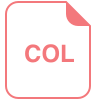
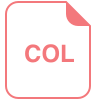
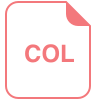
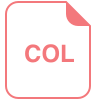
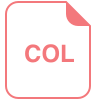
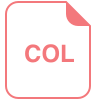
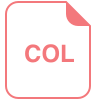