已知E盘根目录存有一音频文件:mymusic.mp3。 编写程序,要求将该文件数据复制到E盘根目录的mylove.mp3文件中。 说明:用字节流和缓冲流两种办法实现。
时间: 2024-03-13 12:47:40 浏览: 17
使用字节流实现:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
String sourceFilePath = "E:\\mymusic.mp3";
String targetFilePath = "E:\\mylove.mp3";
FileInputStream fis = null;
FileOutputStream fos = null;
try {
fis = new FileInputStream(sourceFilePath);
fos = new FileOutputStream(targetFilePath);
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) > 0) {
fos.write(buffer, 0, length);
}
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (fis != null) {
fis.close();
}
if (fos != null) {
fos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
使用缓冲流实现:
```java
import java.io.*;
public class FileCopy {
public static void main(String[] args) {
String sourceFilePath = "E:\\mymusic.mp3";
String targetFilePath = "E:\\mylove.mp3";
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
bis = new BufferedInputStream(new FileInputStream(sourceFilePath));
bos = new BufferedOutputStream(new FileOutputStream(targetFilePath));
byte[] buffer = new byte[1024];
int length;
while ((length = bis.read(buffer)) > 0) {
bos.write(buffer, 0, length);
}
System.out.println("文件复制成功!");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if (bis != null) {
bis.close();
}
if (bos != null) {
bos.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
相关推荐
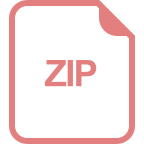














