java获取多层相同JSON数据的所有数据,以集合进行输出,详细代码
时间: 2024-01-21 09:15:57 浏览: 135
假设我们有以下的 JSON 数据:
```json
{
"data": [
{
"id": 1,
"name": "Alice",
"children": [
{
"id": 2,
"name": "Bob",
"children": [
{
"id": 3,
"name": "Charlie",
"children": [
{
"id": 4,
"name": "David",
"children": []
},
{
"id": 5,
"name": "Emily",
"children": []
}
]
}
]
}
]
}
]
}
```
我们要获取所有的 `id` 和 `name`,以集合方式输出。可以用递归的方式来解决这个问题。下面是 Java 代码的实现:
```java
import com.alibaba.fastjson.JSONArray;
import com.alibaba.fastjson.JSONObject;
import java.util.ArrayList;
import java.util.List;
public class Main {
public static void main(String[] args) {
String jsonStr = "{\n" +
" \"data\": [\n" +
" {\n" +
" \"id\": 1,\n" +
" \"name\": \"Alice\",\n" +
" \"children\": [\n" +
" {\n" +
" \"id\": 2,\n" +
" \"name\": \"Bob\",\n" +
" \"children\": [\n" +
" {\n" +
" \"id\": 3,\n" +
" \"name\": \"Charlie\",\n" +
" \"children\": [\n" +
" {\n" +
" \"id\": 4,\n" +
" \"name\": \"David\",\n" +
" \"children\": []\n" +
" },\n" +
" {\n" +
" \"id\": 5,\n" +
" \"name\": \"Emily\",\n" +
" \"children\": []\n" +
" }\n" +
" ]\n" +
" }\n" +
" ]\n" +
" }\n" +
" ]\n" +
" }\n" +
" ]\n" +
"}";
JSONObject jsonObj = JSONObject.parseObject(jsonStr);
List<JSONObject> result = new ArrayList<>();
getAllIdsAndNames(jsonObj.getJSONArray("data"), result);
System.out.println(result);
}
private static void getAllIdsAndNames(JSONArray jsonArray, List<JSONObject> result) {
for (int i = 0; i < jsonArray.size(); i++) {
JSONObject obj = jsonArray.getJSONObject(i);
JSONObject newObj = new JSONObject();
newObj.put("id", obj.getInteger("id"));
newObj.put("name", obj.getString("name"));
result.add(newObj);
JSONArray children = obj.getJSONArray("children");
if (children != null && children.size() > 0) {
getAllIdsAndNames(children, result);
}
}
}
}
```
输出结果为:
```
[{"id":1,"name":"Alice"},{"id":2,"name":"Bob"},{"id":3,"name":"Charlie"},{"id":4,"name":"David"},{"id":5,"name":"Emily"}]
```
阅读全文
相关推荐
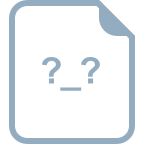
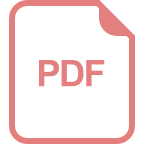
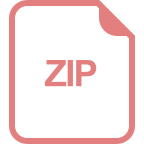
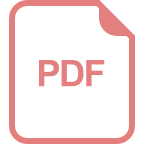
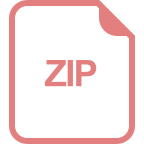
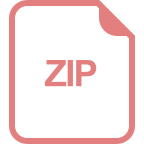
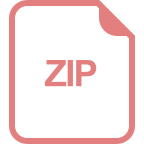
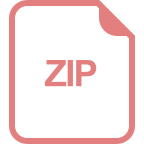
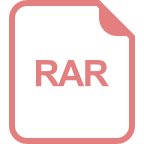
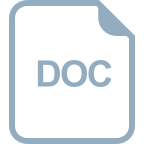
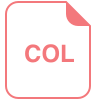
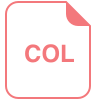
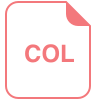
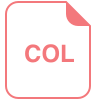
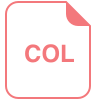


