C语言的while循环简单例题
时间: 2023-06-25 12:06:40 浏览: 69
以下是一个简单的C语言while循环的例题:
```
#include <stdio.h>
int main() {
int num = 1;
while (num <= 10) {
printf("%d\n", num);
num++;
}
return 0;
}
```
这个程序会输出数字1到10,因为while循环的条件是`num <= 10`,只要`num`小于或等于10,就会一直循环。在每次循环中,程序会打印`num`的值,然后将`num`加1,直到`num`等于11时,循环停止。
相关问题
C语言 while循环
C语言中的while循环是一种基本的循环结构,它的语法格式如下:
```
while (condition) {
statement(s);
}
```
其中,condition是循环条件,如果满足条件,则执行循环体中的语句,否则跳出循环。循环体中的语句可以是单个语句或多个语句的复合语句。
下面是一个简单的while循环的例子,它会输出1到10之间的所有整数:
```c
#include <stdio.h>
int main() {
int i = 1;
while (i <= 10) {
printf("%d ", i);
i++;
}
return 0;
}
```
在while循环中,还有两个关键字可以用于控制循环的执行流程,分别是continue和break。其中,continue用于终止本次循环,直接进入下一次循环;而break用于永久的终止循环,直接跳出循环体。
下面是一个使用continue和break的while循环的例子:
```c
#include <stdio.h>
int main() {
int i = 1;
while (i <= 10) {
if (i == 5) {
i++;
continue;
}
if (i == 8) {
break;
}
printf("%d ", i);
i++;
}
return 0;
}
```
在上面的例子中,当i等于5时,使用continue跳过本次循环,直接进入下一次循环;当i等于8时,使用break跳出循环体,结束循环。
c语言双向循环链表例题
以下是一个C语言的双向循环链表的例题:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
struct Node* prev;
};
void insert(struct Node** head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
if (*head == NULL) {
newNode->next = newNode;
newNode->prev = newNode;
*head = newNode;
} else {
struct Node* tail = (*head)->prev;
newNode->next = *head;
(*head)->prev = newNode;
newNode->prev = tail;
tail->next = newNode;
}
}
void display(struct Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
struct Node* current = head;
do {
printf("%d ", current->data);
current = current->next;
} while (current != head);
printf("\n");
}
int main() {
struct Node* head = NULL;
// 插入节点
insert(&head, 1);
insert(&head, 2);
insert(&head, 3);
// 显示链表
display(head);
return 0;
}
```
这个例题演示了如何创建并打印一个双向循环链表。首先,我们创建了一个结构体 `Node`,其中包含数据 `data`、下一个节点指针 `next` 和前一个节点指针 `prev`。然后,我们定义了一个插入函数 `insert`,用于将新节点插入到链表中。最后,我们定义了一个显示函数 `display`,用于打印链表中的所有节点。在 `main` 函数中,我们创建一个空链表,然后插入了三个节点,并使用 `display` 函数打印链表的内容。
这个例题只是对双向循环链表的基本操作进行了演示,你可以根据需要修改和扩展这个代码来实现更复杂的功能。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [链表图解(双向、循环链表+链表增删)](https://blog.csdn.net/qq_52189899/article/details/121552785)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
相关推荐
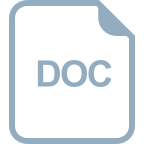
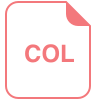
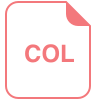
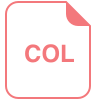
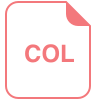
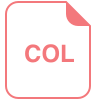







