通过c语言,以栈链的方式将十进制数转换为二进制和八进制 点击复制后,将打开C知道体验页
时间: 2024-05-05 10:16:30 浏览: 98
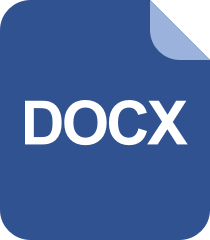
用C语言顺序栈实现十进制和二进制的转换.docx
抱歉,作为AI语言模型,我无法执行代码或打开C知道体验页,但我可以为您提供C语言代码示例。
以下是使用栈链的方式将十进制数转换为二进制和八进制的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node{
int data;
struct node *next;
} Node;
void push(Node **top, int data) {
Node *newNode = (Node*) malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed\n");
return;
}
newNode->data = data;
newNode->next = *top;
*top = newNode;
}
int pop(Node **top) {
if (*top == NULL) {
printf("Stack underflow\n");
return -1;
}
int data = (*top)->data;
Node *temp = *top;
*top = (*top)->next;
free(temp);
return data;
}
void convertToBinary(int decimal) {
Node *stack = NULL;
while (decimal > 0) {
push(&stack, decimal % 2);
decimal /= 2;
}
printf("Binary equivalent: ");
while (stack != NULL) {
printf("%d", pop(&stack));
}
printf("\n");
}
void convertToOctal(int decimal) {
Node *stack = NULL;
while (decimal > 0) {
push(&stack, decimal % 8);
decimal /= 8;
}
printf("Octal equivalent: ");
while (stack != NULL) {
printf("%d", pop(&stack));
}
printf("\n");
}
int main() {
int decimal;
printf("Enter a decimal number: ");
scanf("%d", &decimal);
convertToBinary(decimal);
convertToOctal(decimal);
return 0;
}
```
该程序首先定义了一个节点结构体,用于表示栈链中的节点。然后定义了三个函数:push用于将数据压入栈中,pop用于从栈中弹出数据,convertToBinary和convertToOctal分别用于将十进制数转换为二进制和八进制数。
在convertToBinary和convertToOctal函数中,程序使用while循环将十进制数转换为二进制或八进制数,并压入栈中。然后使用另一个while循环从栈中弹出数据,并输出转换后的二进制或八进制数。
最后,在main函数中,程序从用户输入中获取一个十进制数,并调用convertToBinary和convertToOctal函数将其转换为二进制和八进制数,并输出结果。
该程序使用栈链的方式将十进制数转换为二进制和八进制,具有可读性强、可扩展性好等优点。
阅读全文
相关推荐



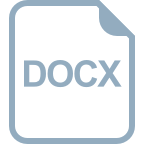
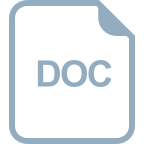









