js websocket 的用法
时间: 2023-11-26 11:05:31 浏览: 47
WebSocket 是一种在单个 TCP 连接上进行全双工通信的协议。使用 WebSocket,服务器可以向客户端推送信息,而不需要客户端轮询服务器来获取信息。在 JavaScript 中使用 WebSocket 可以通过创建 WebSocket 对象,并指定要连接的 WebSocket 服务器的 URL 地址来完成。
相关问题
nestjs websocket 使用方法
NestJS是一个用于构建高效、可靠和可扩展的服务器端应用程序的框架。它使用TypeScript(但也兼容纯JavaScript)构建,遵循模块化的架构。
在NestJS中,WebSocket是一种支持持久连接的网络通信协议。在WebSocket的实现中,服务器和客户端可以相互推送信息,而不需要客户端先发起请求。这使得WebSocket非常适合需要实时双向通信的应用程序。
以下是使用NestJS中WebSocket的基本步骤:
1. 安装依赖:首先,你需要安装`@nestjs/websockets`和`socket.io`这两个包来使用WebSocket功能。
```bash
npm install @nestjs/websockets socket.io
```
2. 创建WebSocket模块:你可以使用NestJS CLI命令来快速创建一个WebSocket模块。
```bash
nest g module websockets
nest g service websockets
```
3. 定义消息代理:在WebSocket模块中,你可以定义消息代理(message handler),这与HTTP请求处理的控制器类似。消息代理处理来自客户端的特定事件,并可将消息发送回客户端。
```typescript
// websockets.service.ts
import { Injectable } from '@nestjs/common';
import { Server, WsResponse } from '@nestjs/websockets';
@Injectable()
export class WebsocketsService {
@WsResponse()
public readonly response = {};
@Server('ws')
onModuleInit(server: Server) {
server.on('connection', (client: any) => {
console.log('Client connected', client.id);
});
}
@EventPattern('message')
async handleTextMessage(client: any, data: string): Promise<void> {
client.emit('message', 'Server received message: ' + data);
}
@EventPattern('disconnect')
onDisconnect(client: any): void {
console.log('Client disconnected', client.id);
}
}
```
4. 使用WebSocket模块:最后,你需要在你的应用模块中导入并使用这个WebSocket模块。
```typescript
// app.module.ts
import { Module } from '@nestjs/common';
import { AppController } from './app.controller';
import { AppService } from './app.service';
import { WebsocketsModule } from './websockets/websockets.module';
@Module({
imports: [WebsocketsModule],
controllers: [AppController],
providers: [AppService],
})
export class AppModule {}
```
现在,你的NestJS应用已经能够处理WebSocket连接和消息了。
websocket使用方法
WebSocket 是一种全双工通信协议,它可以在客户端与服务器之间进行双向通信。
使用 WebSocket 的方法如下:
1. 服务器端配置:在服务器端配置 WebSocket 服务器,并监听指定的端口。
2. 客户端连接:在客户端,使用 WebSocket API 创建一个 WebSocket 对象并连接到服务器端。
3. 发送数据:在客户端和服务器端,通过 WebSocket 对象的 send() 方法来发送数据。
4. 接收数据:在客户端和服务器端,通过 WebSocket 对象的 onmessage 事件来接收数据。
5. 关闭连接:在客户端或服务器端,通过调用 WebSocket 对象的 close() 方法来关闭连接。
WebSocket 可以使用不同的编程语言(例如 JavaScript、Java、Python 等)实现,具体的实现方式可以参考相关语言的文档。
阅读全文
相关推荐
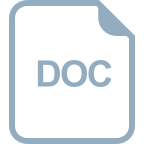
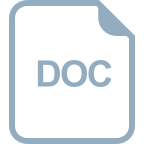
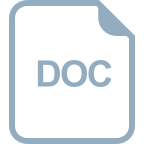
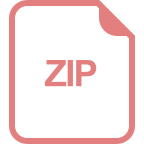
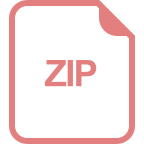
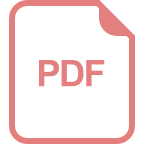
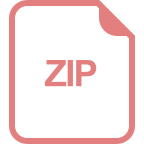








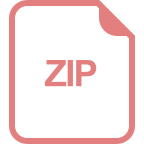
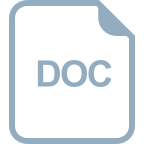