神经网络解决鸢尾花数的代码
时间: 2023-06-04 13:06:59 浏览: 120
以下是解决鸢尾花数据集的神经网络代码示例:
```python
import numpy as np
from sklearn.datasets import load_iris
# 导入数据集
iris = load_iris()
# 提取特征和目标变量
X = iris.data
y = iris.target
# 将目标变量转换为 one-hot 编码
y_onehot = np.zeros((len(y), 3))
y_onehot[np.arange(len(y)), y] = 1
# 定义神经网络模型
class NeuralNetwork:
def __init__(self, input_dim, hidden_dim, output_dim):
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.output_dim = output_dim
self.W1 = np.random.randn(input_dim, hidden_dim) / np.sqrt(input_dim)
self.b1 = np.zeros((1, hidden_dim))
self.W2 = np.random.randn(hidden_dim, output_dim) / np.sqrt(hidden_dim)
self.b2 = np.zeros((1, output_dim))
def forward(self, X):
self.z1 = np.dot(X, self.W1) + self.b1
self.a1 = np.tanh(self.z1)
self.z2 = np.dot(self.a1, self.W2) + self.b2
exp_scores = np.exp(self.z2)
return exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
def backward(self, X, y, output):
delta3 = output - y
dW2 = np.dot(self.a1.T, delta3)
db2 = np.sum(delta3, axis=0, keepdims=True)
delta2 = np.dot(delta3, self.W2.T) * (1 - np.power(self.a1, 2))
dW1 = np.dot(X.T, delta2)
db1 = np.sum(delta2, axis=0)
return dW1, db1, dW2, db2
def train(self, X, y, num_passes=20000, eta=0.01):
for i in range(num_passes):
output = self.forward(X)
dW1, db1, dW2, db2 = self.backward(X, y, output)
self.W1 += -eta * dW1
self.b1 += -eta * db1
self.W2 += -eta * dW2
self.b2 += -eta * db2
# 训练神经网络模型
input_dim = X.shape[1]
hidden_dim = 10
output_dim = 3
nn = NeuralNetwork(input_dim, hidden_dim, output_dim)
nn.train(X, y_onehot)
# 使用训练好的神经网络模型进行预测
y_pred = np.argmax(nn.forward(X), axis=1)
accuracy = np.mean(y_pred == y)
print("训练集准确率:", accuracy)
```
请注意,上面的代码只是示例,可以根据需要进行修改和优化。
阅读全文
相关推荐
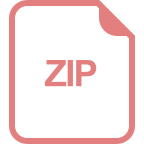
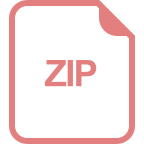
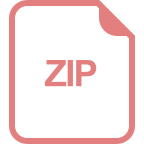
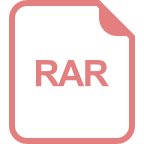
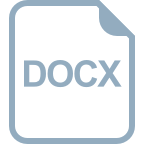
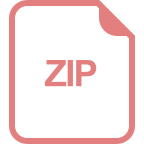
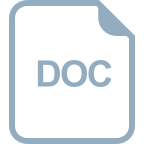
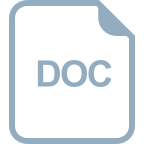
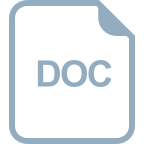
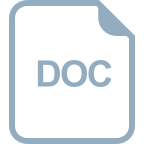





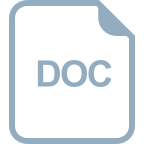
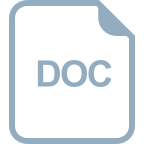
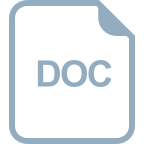