用Java,jsp,mybatis,springmvc,mysql写一个可增删改查的留言版
时间: 2023-12-23 12:03:18 浏览: 241
好的,这是一个比较全面的项目,需要多个技术栈的综合运用。下面是一个简单的留言板的实现步骤:
1. 创建数据库表
首先需要在MySQL中创建一个留言表,包含留言ID、留言标题、留言内容、留言时间等字段。可以参考以下SQL语句:
```
CREATE TABLE `message` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(50) NOT NULL COMMENT '留言标题',
`content` varchar(500) NOT NULL COMMENT '留言内容',
`create_time` datetime NOT NULL COMMENT '留言时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8 COMMENT='留言表';
```
2. 配置Mybatis
在Mybatis的配置文件中,需要定义数据源和Mapper接口。
数据源的配置可以使用Spring提供的DataSource,也可以使用Mybatis提供的PooledDataSource。这里以使用Spring的DataSource为例:
```
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="{driverClassName}" />
<property name="url" value="{url}" />
<property name="username" value="{username}" />
<property name="password" value="{password}" />
</bean>
```
Mapper接口需要定义留言的增删改查操作,可以参考以下示例:
```
public interface MessageMapper {
void insert(Message message);
void delete(Integer id);
void update(Message message);
List<Message> selectAll();
}
```
3. 配置Spring MVC
在Spring MVC的配置文件中,需要定义Controller和视图解析器。
Controller可以根据请求路径和请求方法来定义,可以参考以下示例:
```
@Controller
@RequestMapping("/message")
public class MessageController {
@Autowired
private MessageService messageService;
@ResponseBody
@RequestMapping(value = "/add", method = RequestMethod.POST)
public String add(@RequestBody Message message) {
messageService.add(message);
return "success";
}
@ResponseBody
@RequestMapping(value = "/delete", method = RequestMethod.POST)
public String delete(@RequestParam Integer id) {
messageService.delete(id);
return "success";
}
@ResponseBody
@RequestMapping(value = "/update", method = RequestMethod.POST)
public String update(@RequestBody Message message) {
messageService.update(message);
return "success";
}
@ResponseBody
@RequestMapping(value = "/list", method = RequestMethod.GET)
public List<Message> list() {
return messageService.list();
}
}
```
视图解析器可以根据请求路径和请求方法来定义,可以参考以下示例:
```
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/views/" />
<property name="suffix" value=".jsp" />
</bean>
```
4. 编写JSP页面
在JSP页面中,可以通过AJAX来发送请求和接收响应。可以参考以下示例:
```
<script>
function addMessage() {
var title = $('#title').val();
var content = $('#content').val();
$.ajax({
url: '/message/add',
type: 'POST',
data: JSON.stringify({title: title, content: content}),
contentType: 'application/json',
success: function (data) {
alert('留言成功');
window.location.reload();
}
});
}
function deleteMessage(id) {
$.ajax({
url: '/message/delete',
type: 'POST',
data: {id: id},
success: function (data) {
alert('删除成功');
window.location.reload();
}
});
}
function updateMessage(id) {
var title = $('#title-' + id).val();
var content = $('#content-' + id).val();
$.ajax({
url: '/message/update',
type: 'POST',
data: JSON.stringify({id: id, title: title, content: content}),
contentType: 'application/json',
success: function (data) {
alert('修改成功');
window.location.reload();
}
});
}
</script>
<table>
<thead>
<tr>
<th>ID</th>
<th>标题</th>
<th>内容</th>
<th>时间</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${messages}" var="message">
<tr>
<td>${message.id}</td>
<td><input type="text" id="title-${message.id}" value="${message.title}"></td>
<td><input type="text" id="content-${message.id}" value="${message.content}"></td>
<td>${message.createTime}</td>
<td>
<button onclick="deleteMessage(${message.id})">删除</button>
<button onclick="updateMessage(${message.id})">修改</button>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<form>
<p>标题:<input type="text" id="title"></p>
<p>内容:<input type="text" id="content"></p>
<button type="button" onclick="addMessage()">提交</button>
</form>
```
这样一个简单的增删改查的留言板就完成了。当然,这只是一个简单的示例,实际项目中还需要考虑异常处理、分页查询、安全性等问题。
阅读全文
相关推荐
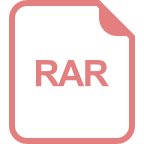
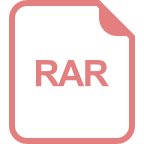
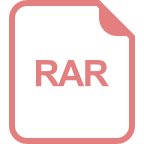















