电脑病毒检测程序 C语言
时间: 2024-01-12 22:04:19 浏览: 164
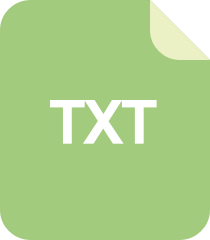
C语言编写的病毒范例
好的,您需要一个电脑病毒检测程序,可以使用C语言来实现。下面是一个简单的示例程序,用于检测指定文件夹中的所有文件是否包含病毒:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_PATH 260 // 文件路径最大长度
#define MAX_VIRUS_NAME 50 // 病毒名称最大长度
// 病毒特征码
char virus_signature[] = "This is a virus!";
// 检查文件是否包含病毒
int check_file(const char *file_path) {
FILE *fp;
char buffer[1024];
int found = 0;
fp = fopen(file_path, "rb");
if (fp == NULL) {
fprintf(stderr, "Could not open file %s\n", file_path);
return 0;
}
while (!feof(fp)) {
size_t size = fread(buffer, 1, sizeof(buffer), fp);
if (size > 0) {
if (strstr(buffer, virus_signature) != NULL) {
found = 1;
break;
}
}
}
fclose(fp);
return found;
}
// 遍历文件夹
void traverse_folder(const char *folder_path) {
char path[MAX_PATH];
char virus_name[MAX_VIRUS_NAME];
int virus_count = 0;
sprintf(virus_name, "virus_%d.txt", rand());
// 打开文件记录病毒名单
FILE *virus_file = fopen(virus_name, "w");
if (virus_file == NULL) {
fprintf(stderr, "Could not create virus file\n");
return;
}
// 遍历文件夹
DIR *dir;
struct dirent *ent;
if ((dir = opendir(folder_path)) != NULL) {
while ((ent = readdir(dir)) != NULL) {
if (ent->d_type == DT_REG) {
sprintf(path, "%s/%s", folder_path, ent->d_name);
if (check_file(path)) {
fprintf(virus_file, "%s\n", path);
virus_count++;
}
}
}
closedir(dir);
} else {
fprintf(stderr, "Could not open directory %s\n", folder_path);
}
fclose(virus_file);
printf("%d viruses found. Check %s for details.\n", virus_count, virus_name);
}
int main(int argc, char **argv) {
if (argc < 2) {
fprintf(stderr, "Usage: %s <folder>\n", argv[0]);
return 1;
}
srand((unsigned int)time(NULL)); // 初始化随机数种子
traverse_folder(argv[1]);
return 0;
}
```
该程序使用了一个简单的病毒特征码来检查文件是否包含病毒,如果发现病毒,则将文件路径记录到一个文件中。您可以根据需要修改特征码和病毒名单文件的名称。另外,该程序只检查指定文件夹中的普通文件,不包括子文件夹和其他类型的文件。如果需要检查所有类型的文件,可以根据需要进行修改。
阅读全文
相关推荐
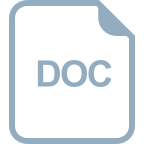
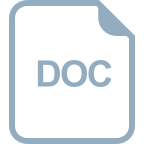
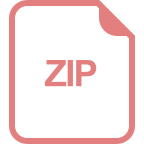
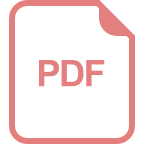
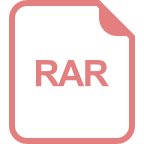
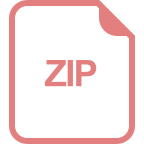
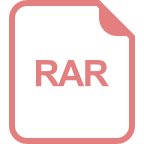
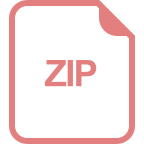
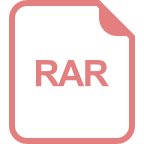
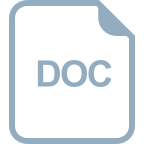
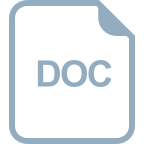
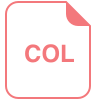
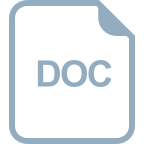
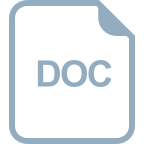
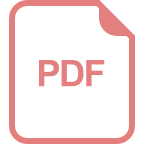
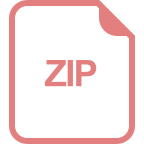
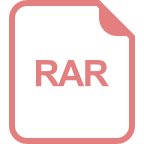
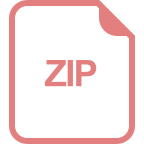