使用PHP语言连接数据库,写一个登陆功能,带图形验证码
时间: 2024-03-18 15:38:40 浏览: 65
好的,我可以帮你回答这个问题。首先,你需要在 PHP 中连接数据库。以下是一个示例代码,你需要将其替换为你的数据库信息:
```php
<?php
$servername = "localhost";
$username = "yourusername";
$password = "yourpassword";
$dbname = "yourdatabasename";
// 创建连接
$conn = new mysqli($servername, $username, $password, $dbname);
// 检查连接是否成功
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
echo "Connected successfully";
?>
```
接下来,你需要创建一个包含表单的登录页面。以下是一个示例代码,包含了一个图形验证码:
```php
<?php
session_start();
if(isset($_POST['submit'])) {
// 检查验证码是否正确
if($_SESSION['captcha'] != $_POST['captcha']) {
echo "验证码错误";
} else {
// 获取表单数据
$username = $_POST['username'];
$password = $_POST['password'];
// 查询数据库
$sql = "SELECT * FROM users WHERE username='$username' AND password='$password'";
$result = $conn->query($sql);
// 检查用户名和密码是否匹配
if ($result->num_rows > 0) {
echo "登录成功";
} else {
echo "用户名或密码错误";
}
}
}
?>
<form method="post" action="">
<label>用户名:</label>
<input type="text" name="username" required><br><br>
<label>密码:</label>
<input type="password" name="password" required><br><br>
<label>验证码:</label>
<input type="text" name="captcha" required>
<img src="captcha.php" alt="验证码"><br><br>
<input type="submit" name="submit" value="登录">
</form>
```
在上面的代码中,我们使用了一个名为 captcha.php 的文件来生成图形验证码。以下是该文件的示例代码:
```php
<?php
session_start();
header('Content-type: image/png');
// 生成验证码
$captcha = substr(md5(rand()), 0, 7);
// 保存验证码到 session
$_SESSION['captcha'] = $captcha;
// 创建图像
$im = imagecreatetruecolor(80, 30);
// 设置背景色和文本色
$bgColor = imagecolorallocate($im, 255, 255, 255);
$textColor = imagecolorallocate($im, 0, 0, 0);
// 填充背景色
imagefill($im, 0, 0, $bgColor);
// 添加文本
imagestring($im, 5, 20, 8, $captcha, $textColor);
// 输出图像
imagepng($im);
// 释放内存
imagedestroy($im);
?>
```
最后,你需要将上述代码保存为 PHP 文件并上传到你的服务器上。注意,你需要将数据库信息替换为你自己的信息,并根据需要更改表格和字段名。
阅读全文
相关推荐
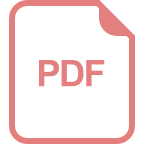
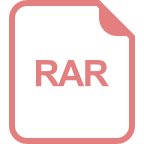
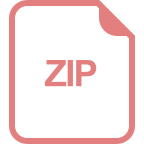
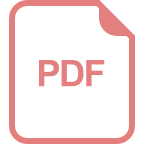
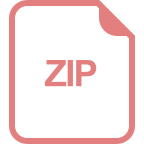
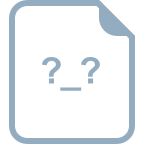
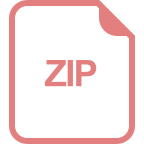
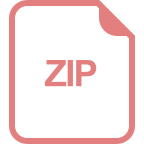
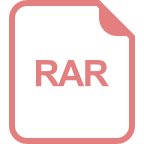
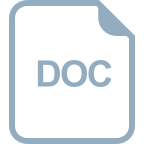
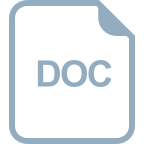
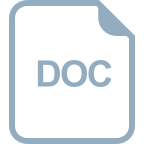
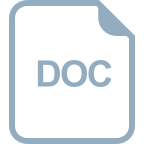
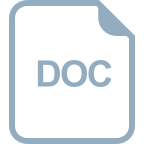
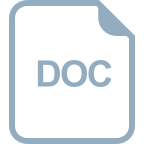
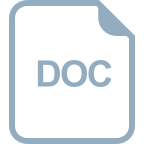