定时任务定期维护异常的游戏渠道关联关系数,把不用的渠道游戏关联关系移动到备份表,游戏状态通过后再移到原渠道游戏关联关系表测试用例
时间: 2023-08-16 21:05:46 浏览: 39
1. 编写定时任务程序,可以使用 Python 的第三方库 APScheduler 来实现定时任务功能,具体实现方法如下:
```python
from apscheduler.schedulers.blocking import BlockingScheduler
def job():
# 这里编写定时任务要执行的代码
pass
scheduler = BlockingScheduler()
scheduler.add_job(job, 'interval', hours=24) # 每 24 小时执行一次任务
scheduler.start()
```
2. 编写异常游戏渠道关联关系维护程序,该程序需要连接游戏数据库,查询游戏渠道关联关系表中状态异常的数据,将不用的关联关系移动到备份表,并将关联关系状态修改为待测试状态。
```python
import pymysql
# 连接数据库
db = pymysql.connect(host='localhost', user='root', password='password', database='game')
# 查询异常数据
cursor = db.cursor()
sql = 'SELECT * FROM channel_game_relation WHERE status != "normal"'
cursor.execute(sql)
results = cursor.fetchall()
# 移动数据到备份表,并修改状态
backup_sql = 'INSERT INTO channel_game_relation_backup (channel_id, game_id, status) VALUES (%s, %s, %s)'
update_sql = 'UPDATE channel_game_relation SET status="testing" WHERE channel_id=%s AND game_id=%s'
for result in results:
channel_id, game_id, status = result
cursor.execute(backup_sql, (channel_id, game_id, status))
cursor.execute(update_sql, (channel_id, game_id))
db.commit()
db.close()
```
3. 编写测试程序,该程序需要连接游戏数据库,查询备份表中状态为待测试的数据,进行测试后将数据移动回原渠道游戏关联关系表。
```python
import pymysql
# 连接数据库
db = pymysql.connect(host='localhost', user='root', password='password', database='game')
# 查询待测试数据
cursor = db.cursor()
sql = 'SELECT * FROM channel_game_relation_backup WHERE status="testing"'
cursor.execute(sql)
results = cursor.fetchall()
# 进行测试
test_sql = 'SELECT * FROM test_table WHERE channel_id=%s AND game_id=%s'
for result in results:
channel_id, game_id, status = result
cursor.execute(test_sql, (channel_id, game_id))
test_result = cursor.fetchone()
# 如果测试通过,将数据移动回原渠道游戏关联关系表
if test_result == 'pass':
insert_sql = 'INSERT INTO channel_game_relation (channel_id, game_id, status) VALUES (%s, %s, %s)'
delete_sql = 'DELETE FROM channel_game_relation_backup WHERE channel_id=%s AND game_id=%s'
cursor.execute(insert_sql, (channel_id, game_id, 'normal'))
cursor.execute(delete_sql, (channel_id, game_id))
db.commit()
db.close()
```
4. 编写测试用例,包括对定时任务程序、异常游戏渠道关联关系维护程序和测试程序的功能进行测试,确保功能正确性。
相关推荐
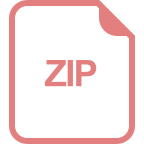
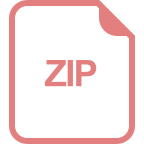















