编写一段程序,使用系统调用fork( )创建一个子进程,在子进程中显示该子进程及其父进程的PID,然后子进程睡眠10秒钟(使用系统调用sleep(10));父进程通过调用wait( )等待子进程结束,并显示该子进程的PID。
时间: 2023-05-24 16:06:09 浏览: 81
```c
#include <unistd.h>
#include <stdio.h>
#include <stdlib.h>
#include <sys/wait.h>
int main()
{
pid_t pid;
pid = fork(); // 创建子进程
if (pid < 0) // 创建子进程失败
{
fprintf(stderr, "Error: fork() failed.\n");
exit(1);
}
else if (pid == 0) // 子进程
{
printf("子进程PID:%d,父进程PID:%d\n", getpid(), getppid()); // 显示子进程和父进程的PID
sleep(10); // 子进程睡眠10秒钟
exit(0); // 子进程结束
}
else // 父进程
{
int status;
wait(&status); // 父进程通过wait()等待子进程结束
printf("子进程PID:%d 已结束\n", pid); // 显示子进程的PID
}
return 0;
}
```
相关问题
编写一段程序,使用系统调用fork( )创建一个子进程,在子进程中显示该子进程及其父进程的pid,然后子进程睡眠10秒钟(使用系统调用sleep(10));父进程通过调用wait( )等待子进程结束,并显示该子进程的pid
好的,以下是代码实现:
```c++
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid;
pid = fork(); // 创建子进程
if (pid < 0) { // 创建失败
printf("Fork error!\n");
return 1;
} else if (pid == 0) { // 子进程
printf("This is child process, PID is %d, parent PID is %d.\n", getpid(), getppid());
sleep(10); // 子进程睡眠10秒钟
} else { // 父进程
printf("This is parent process, child PID is %d.\n", pid);
wait(NULL); // 等待子进程结束
}
return 0;
}
```
在上面的代码中,我们使用了 `fork()` 系统调用创建了一个子进程,并通过 `getpid()` 和 `getppid()` 获取了子进程和父进程的PID。然后在子进程中使用了 `sleep(10)` 系统调用让子进程睡眠10秒钟。在父进程中使用了 `wait(NULL)` 等待子进程结束,并通过 `pid` 变量获取了子进程的PID。
希望这个程序能够帮助你理解 `fork()` 和 `wait()` 系统调用的使用。如果你还有其他问题,请继续提问。
)编写一段程序,使用系统调用fork()创建一个子进程。在子进程中,用系统调用 getid()获得子进程TD。在父进程中,通过系统调用 wait()来阻塞白己,并等待收集子进程的进程ID,把它彻底销毁后返回。
在Linux或Unix系统中,你可以使用C语言的标准库函数`fork()`、`waitpid()`和`gettid()`来完成这个任务。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t child_pid; // 子进程PID
tid_t child_tid; // 子进程TID
// 创建子进程
if ((child_pid = fork()) == -1) {
perror("Fork failed");
return 1;
}
if (child_pid == 0) { // 子进程
// 在子进程中获取TID
child_tid = syscall(SYS_gettid);
printf("Child process with PID %d and TID %d\n", getpid(), child_tid);
// 父进程需要等待子进程结束
} else { // 父进程
// 阻塞并等待子进程结束
int status;
pid_t result = waitpid(child_pid, &status, 0);
if (result == -1) {
perror("Waitpid failed");
return 1;
}
printf("Parent process received the exit status of child: %d\n", WIFEXITED(status) ? WEXITSTATUS(status) : WIFSIGNALED(status));
printf("Child with PID %d has been terminated.\n", child_pid);
}
return 0;
}
```
在这个程序中,`fork()`用于创建子进程,如果返回0,则表示当前进程是新创建的子进程;否则返回的是子进程的PID。然后,在子进程中,我们使用`syscall(SYS_gettid)`获取当前线程的TID。
父进程则通过`waitpid()`等待子进程结束,当子进程结束后,父进程会接收子进程的状态信息。注意,`waitpid()`函数会阻塞直到子进程结束,并返回其退出状态。
阅读全文
相关推荐
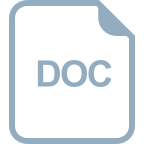
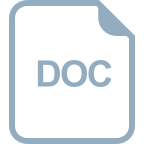
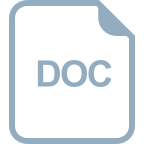









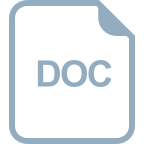
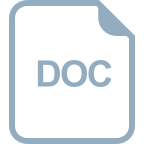
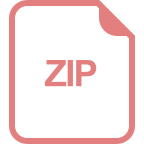
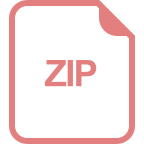
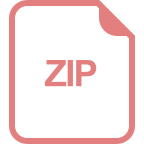
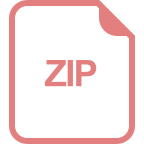