flutter 实现表格合并单元格
时间: 2024-02-01 13:03:30 浏览: 320
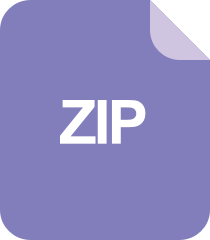
Flex 表格单元格合并

Flutter 目前还没有内置的表格控件,但是可以使用 `Table` 和 `TableCell` 来实现表格合并单元格。
具体实现步骤如下:
1. 定义表格数据和合并单元格的规则。
```dart
List<List<String>> tableData = [
['A1', 'B1', 'C1'],
['A2', 'B2', 'C2'],
['A3', 'B3', 'C3'],
['A4', 'B4', 'C4'],
];
Map<int, List<TableCell>> mergedCells = {
0: [
TableCell(
child: Text('A1'),
rowSpan: 2,
columnSpan: 2,
),
null,
TableCell(
child: Text('C1'),
rowSpan: 2,
),
],
};
```
2. 根据表格数据和合并单元格的规则构建表格控件。
```dart
Table(
children: tableData.asMap().entries.map((row) {
int rowIndex = row.key;
List<String> rowData = row.value;
List<TableCell> cells = rowData.asMap().entries.map((cell) {
int columnIndex = cell.key;
String cellData = cell.value;
if (mergedCells.containsKey(rowIndex) &&
mergedCells[rowIndex][columnIndex] != null) {
// 如果当前单元格需要合并,则返回合并单元格的配置
return mergedCells[rowIndex][columnIndex];
} else {
// 否则返回普通单元格
return TableCell(
child: Text(cellData),
);
}
}).toList();
return TableRow(
children: cells,
);
}).toList(),
)
```
3. 运行程序,即可看到合并单元格的表格效果。
完整代码如下:
```dart
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
List<List<String>> tableData = [
['A1', 'B1', 'C1'],
['A2', 'B2', 'C2'],
['A3', 'B3', 'C3'],
['A4', 'B4', 'C4'],
];
Map<int, List<TableCell>> mergedCells = {
0: [
TableCell(
child: Text('A1'),
rowSpan: 2,
columnSpan: 2,
),
null,
TableCell(
child: Text('C1'),
rowSpan: 2,
),
],
};
return MaterialApp(
title: 'Flutter Demo',
home: Scaffold(
appBar: AppBar(
title: Text('Table with merged cells'),
),
body: Center(
child: Table(
children: tableData.asMap().entries.map((row) {
int rowIndex = row.key;
List<String> rowData = row.value;
List<TableCell> cells = rowData.asMap().entries.map((cell) {
int columnIndex = cell.key;
String cellData = cell.value;
if (mergedCells.containsKey(rowIndex) &&
mergedCells[rowIndex][columnIndex] != null) {
// 如果当前单元格需要合并,则返回合并单元格的配置
return mergedCells[rowIndex][columnIndex];
} else {
// 否则返回普通单元格
return TableCell(
child: Text(cellData),
);
}
}).toList();
return TableRow(
children: cells,
);
}).toList(),
),
),
),
);
}
}
```
阅读全文
相关推荐
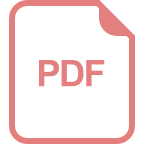






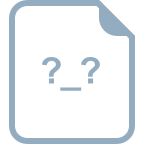
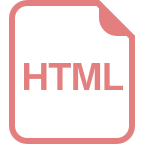
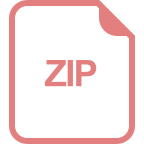
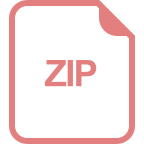