能用c++做一个中文转英文的一个字典互译吗
时间: 2023-08-09 11:08:54 浏览: 404
是的,可以使用C++编写一个中文-英文的字典翻译程序。其中,需要以下步骤:
1. 收集中英文翻译对应关系的数据,可以使用已有的开源数据或自己整理数据。
2. 读取用户输入的中文或英文单词。
3. 使用算法查询翻译对应关系,比如可以使用哈希表或二叉查找树等数据结构。
4. 输出翻译结果。
以下是一个简单的中英文翻译程序示例:
```c++
#include <iostream>
#include <unordered_map>
#include <string>
using namespace std;
int main() {
// 初始化中英文翻译对应关系
unordered_map<string, string> dict = {
{"你好", "Hello"},
{"世界", "World"},
{"好的", "OK"},
{"谢谢", "Thank you"},
{"再见", "Goodbye"}
};
// 读取用户输入
string input;
cout << "请输入中文或英文单词:";
getline(cin, input);
// 查询翻译结果
if (dict.count(input)) {
cout << dict[input] << endl;
} else {
for (const auto& pair : dict) {
if (pair.second == input) {
cout << pair.first << endl;
break;
}
}
}
return 0;
}
```
这个程序使用了C++11中的`unordered_map`容器来存储中英文翻译对应关系,通过`count`函数查询中文或英文单词是否存在于字典中。如果存在,则输出对应的翻译结果;否则,遍历整个字典,输出第一个英文翻译结果与用户输入相同的中文单词。
阅读全文
相关推荐
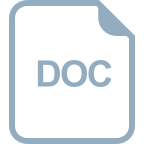
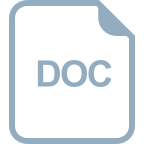
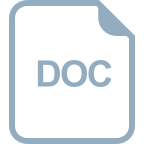
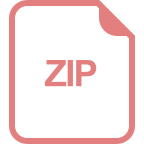


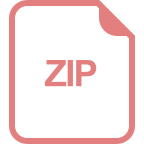
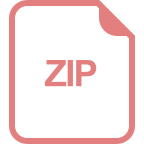
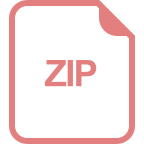
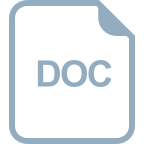

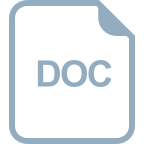
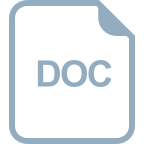
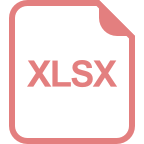
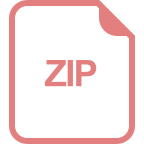
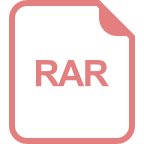
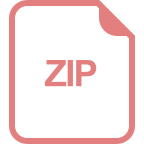
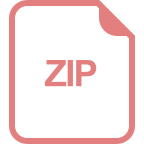
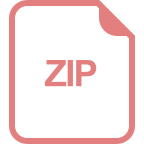
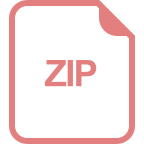