把字符数组中的字母由小到大
时间: 2024-01-31 13:08:28 浏览: 80
好的,可以通过以下的C++代码实现:
```c++
#include <iostream>
#include <cstring>
#include <algorithm>
using namespace std;
int main() {
char str[] = "chitgpt";
int len = strlen(str);
sort(str, str + len);
cout << "排序后的字符串为:" << str << endl;
return 0;
}
```
上述代码中,我们使用了C++的STL库中的sort函数对字符数组进行排序,最终输出排序后的字符串。请注意,这段代码中的输出语句是为了展示排序结果,实际应用中可能不需要输出。
相关问题
编写程序,把字符数组中的字母按由小到大的顺序排列并输出
好的,以下是一个简单的 C++ 程序,可以实现这个功能:
```cpp
#include <iostream>
#include <algorithm>
#include <cstring>
using namespace std;
int main() {
char s[] = "hello world"; // 待排序的字符数组
int n = strlen(s);
sort(s, s + n); // 使用 sort 函数进行排序
cout << s << endl; // 输出排序后的结果
return 0;
}
```
程序中使用了 C++ STL 中的 `sort` 函数,它可以对指定区间的元素进行排序。在这个例子中,我们对整个字符数组进行排序,因此 `sort` 函数的第一个参数为 `s`,第二个参数为 `s + n`,即指向字符数组最后一个元素的下一个位置。排序后,我们直接输出字符数组即可。
把字符数组中的字母按由小到大的顺序排序并输出 ,不用sort函数
好的,这是一个关于算法的问题,可以回答。以下是解决方案:
```cpp
#include <iostream>
using namespace std;
int main()
{
char str[100];
cin >> str;
int count[26] = { 0 };
for (int i = 0; str[i] != '\0'; i++) {
count[str[i] - 'a']++; // 统计每个字母出现的次数
}
for (int i = 0; i < 26; i++) {
for (int j = 0; j < count[i]; j++) {
cout << (char)(i + 'a'); // 输出按由小到大排序的字母
}
}
return 0;
}
```
以上代码中,我们首先定义了一个字符数组 `str`,并通过 `cin` 从标准输入读入待排序的字符串。然后我们定义一个整型数组 `count`,用于统计字符串中每个字母出现的次数。由于小写字母只有 26 个,因此数组大小为 26。
接着,我们遍历字符串中的每个字符,计算该字符在字符串中出现的次数,并将其保存在 `count` 数组中对应位置。然后我们再次遍历 `count` 数组,从小到大输出字符。
这里的关键在于如何统计字符串中每个字母的出现次数。我们可以使用 `count[str[i] - 'a']++` 的方式,在数组 `count` 中对每个字母出现的次数进行累加。由于小写字母的 ASCII 码值是递增的,因此可以用 `str[i] - 'a'` 的方式将每个字符映射到 `count` 数组中的对应位置。
注意,以上代码并没有使用 `sort` 函数,而是通过遍历数组进行排序的。这种算法的时间复杂度是 $O(n)$,属于比较高效的排序算法之一。
阅读全文
相关推荐
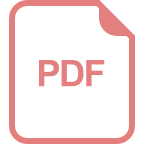
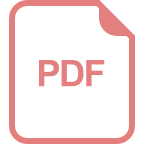
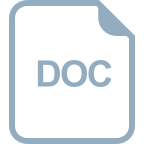













