帮我写一个stm32spi只读模式读取16位ads8320的程序,在读取0后读取16位数据
时间: 2023-08-04 21:07:16 浏览: 148
好的,以下是一个使用STM32的SPI接口读取ADS8320数据的示例程序,其中在读取到0后再读取16位数据:
```c
#include "stm32f4xx.h"
#define ADS8320_CS_PIN GPIO_Pin_0
#define ADS8320_CS_PORT GPIOA
void ADS8320_Init(void)
{
GPIO_InitTypeDef GPIO_InitStruct;
SPI_InitTypeDef SPI_InitStruct;
// Enable GPIO clock
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA, ENABLE);
// Enable SPI clock
RCC_APB1PeriphClockCmd(RCC_APB1Periph_SPI2, ENABLE);
// Configure SPI pins
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_13 | GPIO_Pin_14 | GPIO_Pin_15;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStruct);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource13, GPIO_AF_SPI2);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource14, GPIO_AF_SPI2);
GPIO_PinAFConfig(GPIOB, GPIO_PinSource15, GPIO_AF_SPI2);
// Configure CS pin
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_Pin = ADS8320_CS_PIN;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(ADS8320_CS_PORT, &GPIO_InitStruct);
// Configure SPI
SPI_InitStruct.SPI_Direction = SPI_Direction_2Lines_FullDuplex;
SPI_InitStruct.SPI_DataSize = SPI_DataSize_16b;
SPI_InitStruct.SPI_CPOL = SPI_CPOL_Low;
SPI_InitStruct.SPI_CPHA = SPI_CPHA_1Edge;
SPI_InitStruct.SPI_NSS = SPI_NSS_Soft;
SPI_InitStruct.SPI_BaudRatePrescaler = SPI_BaudRatePrescaler_4;
SPI_InitStruct.SPI_FirstBit = SPI_FirstBit_MSB;
SPI_InitStruct.SPI_Mode = SPI_Mode_Master;
SPI_Init(SPI2, &SPI_InitStruct);
SPI_Cmd(SPI2, ENABLE);
}
void ADS8320_Read(uint16_t *data)
{
// Select ADS8320
GPIO_ResetBits(ADS8320_CS_PORT, ADS8320_CS_PIN);
// Send read command
SPI_I2S_SendData(SPI2, 0x0000);
// Wait for data to be received
while(SPI_I2S_GetFlagStatus(SPI2, SPI_I2S_FLAG_RXNE) == RESET);
// Read data
*data = SPI_I2S_ReceiveData(SPI2);
// Check if the first data is 0
if ((*data & 0xFF00) == 0x0000) {
// Read the second 16-bit data
while(SPI_I2S_GetFlagStatus(SPI2, SPI_I2S_FLAG_RXNE) == RESET);
*data = SPI_I2S_ReceiveData(SPI2);
}
// Deselect ADS8320
GPIO_SetBits(ADS8320_CS_PORT, ADS8320_CS_PIN);
}
int main(void)
{
uint16_t data;
// Initialize ADS8320
ADS8320_Init();
// Read data from ADS8320
ADS8320_Read(&data);
while(1);
}
```
以上代码中,当读取到的第一个16位数据的高8位为0时,说明此时读取到的数据为0,需要再次读取一次,以获取正确的数据。如果第一个数据的高8位不为0,则说明直接读取到了正确的数据。
需要注意的是,以上代码仅供参考,具体实现可能需要根据您的具体硬件和需求进行调整。
相关推荐
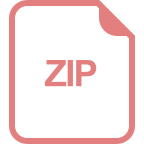
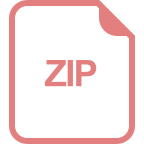














