qml动态创建一个控件
时间: 2023-11-01 14:14:47 浏览: 107
可以使用QML中的Component来动态创建控件。以下是一个简单的例子:
```
import QtQuick 2.0
Rectangle {
width: 200
height: 200
Component {
id: myButtonComponent
Button {
text: "Click me!"
onClicked: console.log("Button clicked!")
}
}
MouseArea {
anchors.fill: parent
onClicked: {
var myButton = myButtonComponent.createObject(parent)
myButton.x = mouseX
myButton.y = mouseY
}
}
}
```
在这个例子中,我们创建了一个Rectangle控件,然后定义了一个Component,其中包含一个Button控件。在MouseArea的onClicked信号处理函数中,我们使用createObject方法创建了一个Button控件实例,并将其添加到父控件中。我们还设置了该控件的位置,以使其出现在鼠标点击的位置。
相关问题
qml要创建很多控件怎么办
如果需要在QtQuick/QML中创建大量控件,可以考虑使用ListView或GridView来进行优化。这两个控件可以动态地生成控件,只在视图内生成,节省了内存并提高了性能。另外,可以在必要时使用Component来创建控件或在必要时对控件进行重用。同时,尽量避免使用较重的控件或控件的数量过多,可以有效减少内存占用和提高性能。
qml 动态添加多个自定义控件
在QML中,你可以使用动态创建组件的方法来添加多个自定义控件。你可以使用Qt.createComponent()函数来动态创建组件实例,并使用createObject()函数来创建组件的多个实例。以下是一个示例代码:
```
import QtQuick 2.0
Rectangle {
id: container
width: 200
height: 200
function createCustomButton() {
var component = Qt.createComponent("CustomButton.qml");
if (component.status === Component.Ready) {
var button = component.createObject(container);
button.x = Math.random() * (container.width - button.width);
button.y = Math.random() * (container.height - button.height);
} else {
console.log("Error creating component:", component.errorString());
}
}
MouseArea {
anchors.fill: parent
onClicked: {
createCustomButton();
}
}
}
```
这个示例中,我们创建了一个名为container的矩形容器,它用于放置自定义按钮。当鼠标点击容器时,会调用createCustomButton()函数来创建一个自定义按钮的实例,并将其放置在容器内的随机位置。你可以根据需要设置按钮的属性和行为。
阅读全文
相关推荐
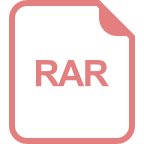
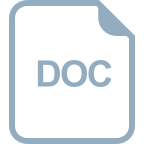






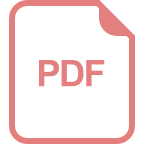
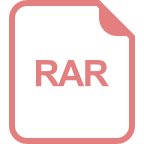
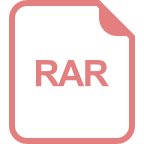
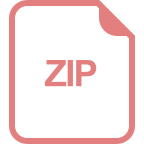
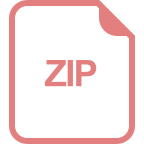
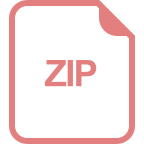