python词频分析系统
时间: 2023-07-26 18:05:42 浏览: 171
Python可以用来实现词频分析系统。以下是一个简单的例子:
```python
import string
from collections import Counter
# 读取文件内容
with open('example.txt', 'r') as f:
text = f.read()
# 去除标点符号和空格
text = text.translate(str.maketrans('', '', string.punctuation + ' '))
text = text.lower()
# 拆分单词
words = text.split()
# 统计单词出现次数
word_counts = Counter(words)
# 输出出现次数最多的前10个单词
for word, count in word_counts.most_common(10):
print(word, count)
```
这个例子会读取名为`example.txt`的文本文件,并统计其中每个单词出现的次数。最后,会输出出现次数最多的前10个单词。你可以根据自己的需求进行修改和扩展。
相关问题
python词频分析系统包含读取文章内容模块、归一化处理模块、去重模块、词频统计模块、指定显示前几名模块、退出程序模块
好的,这个系统可以使用Python编写,包含以下模块:
1. 读取文章内容模块:可以使用Python内置的文件操作函数,例如open()和read()。
2. 归一化处理模块:可以将文章内容转换为小写字母,并去掉标点符号和特殊字符,只留下单词。
3. 去重模块:可以使用Python内置的set()函数,将文章中的单词去重。
4. 词频统计模块:可以使用Python内置的collections模块中的Counter()函数,统计每个单词在文章中出现的次数。
5. 指定显示前几名模块:可以使用Python内置的sorted()函数按照词频进行排序,并选取前几名进行展示。
6. 退出程序模块:可以使用Python内置的sys.exit()函数,退出程序。
下面是一个基本的代码框架:
```python
import string
import collections
import sys
# 读取文章内容模块
def read_file(filename):
with open(filename, 'r') as f:
text = f.read()
return text
# 归一化处理模块
def normalize(text):
text = text.lower()
for c in string.punctuation:
text = text.replace(c, ' ')
return text
# 去重模块
def remove_duplicates(text):
words = text.split()
unique_words = set(words)
return unique_words
# 词频统计模块
def word_count(text):
words = text.split()
word_count = collections.Counter(words)
return word_count
# 指定显示前几名模块
def top_words(word_count, n):
top_words = word_count.most_common(n)
return top_words
# 退出程序模块
def exit_program():
print("程序已退出")
sys.exit()
# 主程序
def main():
# 读取文章内容
filename = "article.txt"
text = read_file(filename)
# 归一化处理
normalized_text = normalize(text)
# 去重
unique_words = remove_duplicates(normalized_text)
# 词频统计
word_count_dict = word_count(normalized_text)
# 指定显示前几名
n = 10
top_words_list = top_words(word_count_dict, n)
# 输出结果
print("文章中出现的单词数:", len(unique_words))
print("文章中出现次数最多的前", n, "个单词:")
for i, word in enumerate(top_words_list):
print(i+1, ". ", word[0], ":", word[1])
# 退出程序
exit_program()
if __name__ == '__main__':
main()
```
python词频统计的应用
Python词频统计在自然语言处理和文本分析中是一种非常常见的应用。以下是一些应用场景:
1. 文本挖掘:通过Python进行词频统计,可以找到文本中最常出现的单词或短语,从而了解文本的主题和关键内容。
2. 情感分析:通过Python进行词频统计,可以找到文本中出现频率最高的情感词汇,如“好”、“坏”等,从而进行情感分析。
3. 推荐系统:通过Python进行词频统计,可以找到用户最常使用的单词或短语,从而提高推荐系统的准确性。
4. 网站SEO优化:通过Python进行词频统计,可以找到网站中最常出现的关键词,从而进行SEO优化,提高网站搜索排名。
在Python中,可以使用NLTK、Jieba等自然语言处理库进行词频统计。例如,使用nltk库进行词频统计的代码如下:
```python
import nltk
from nltk import FreqDist
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 分词
words = nltk.word_tokenize(text)
# 去除停用词
stopwords = nltk.corpus.stopwords.words('english')
words = [word for word in words if word.lower() not in stopwords]
# 统计词频
fdist = FreqDist(words)
# 输出前10个最常出现的单词
print(fdist.most_common(10))
```
阅读全文
相关推荐
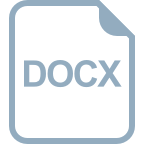
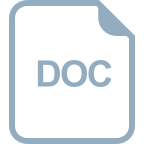
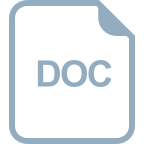
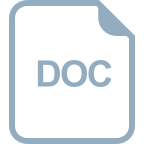
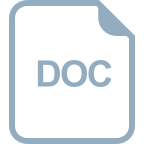
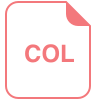


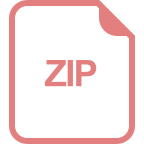
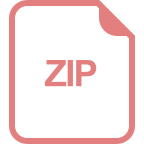
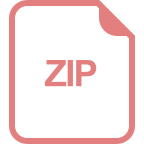
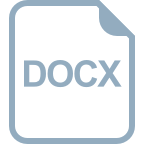
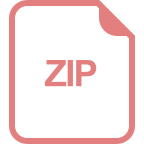
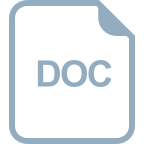