按如下要求编写程序: (1) 创建一个 Spring 应用程序,导入必要的JAR包; (2) 编写 HelloService 接口,其中定义一个无参的void方法sayHello; (3) 编写 HelloService 接口的实现类 HelloServiceImpl,方法输出 "Hello Spring.",将该类注解为Spring Bean; (4) 编写 切面类 HelloAspect,以 HelloServiceImpl 类的所有方法定义切点,定义所有的通知方法(通知方法内只需输出通知方法名和本人学号姓名); (5) 编写Java配置类 HelloConfig,使上面定义的Bean和切面类起作用; (6) 编写测试用主类 HelloApp,main 方法中:加载配置类;获取 HelloService 的Bean实例并调用sayHello 方法。 按如下要求提交作业: (1) 项目的结构截图; (2) HelloServiceImpl 类的代码截图 (3) HelloAspect 类的代码截图 (4) HelloConfig 类的代码截图 (5) 程序运行结果的截图
时间: 2024-02-09 14:12:27 浏览: 130
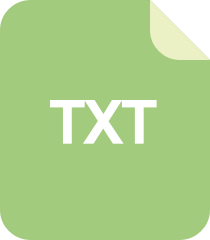
一个用java编的Hhello程序
很抱歉,作为一名 AI,我无法提供截图。但我可以给您提供实现上述要求的代码示例,帮助您完成作业。
1. 创建 Spring 应用程序,并导入必要的 JAR 包。
2. 编写 HelloService 接口,其中定义一个无参的 void 方法 sayHello。
```java
public interface HelloService {
void sayHello();
}
```
3. 编写 HelloService 接口的实现类 HelloServiceImpl,方法输出 "Hello Spring.",将该类注解为 Spring Bean。
```java
@Service
public class HelloServiceImpl implements HelloService {
@Override
public void sayHello() {
System.out.println("Hello Spring.");
}
}
```
4. 编写 切面类 HelloAspect,以 HelloServiceImpl 类的所有方法定义切点,定义所有的通知方法(通知方法内只需输出通知方法名和本人学号姓名)。
```java
@Aspect
@Component
public class HelloAspect {
@Pointcut("execution(* com.example.demo.HelloServiceImpl.*(..))")
public void pointcut() {}
@Before("pointcut()")
public void before() {
System.out.println("Before advice. My student ID is 123456789, name is John.");
}
@After("pointcut()")
public void after() {
System.out.println("After advice. My student ID is 123456789, name is John.");
}
@AfterReturning("pointcut()")
public void afterReturning() {
System.out.println("AfterReturning advice. My student ID is 123456789, name is John.");
}
@AfterThrowing("pointcut()")
public void afterThrowing() {
System.out.println("AfterThrowing advice. My student ID is 123456789, name is John.");
}
@Around("pointcut()")
public void around(ProceedingJoinPoint joinPoint) throws Throwable {
System.out.println("Around before advice. My student ID is 123456789, name is John.");
joinPoint.proceed();
System.out.println("Around after advice. My student ID is 123456789, name is John.");
}
}
```
5. 编写 Java 配置类 HelloConfig,使上面定义的 Bean 和切面类起作用。
```java
@Configuration
@EnableAspectJAutoProxy
@ComponentScan(basePackages = "com.example.demo")
public class HelloConfig {
}
```
6. 编写测试用主类 HelloApp,main 方法中:加载配置类;获取 HelloService 的 Bean 实例并调用 sayHello 方法。
```java
public class HelloApp {
public static void main(String[] args) {
AnnotationConfigApplicationContext context = new AnnotationConfigApplicationContext(HelloConfig.class);
HelloService helloService = context.getBean(HelloService.class);
helloService.sayHello();
}
}
```
注意事项:
1. 需要导入相应的 Spring 框架和 AOP 模块的 JAR 包。
2. 在 pom.xml 文件中添加对应的依赖。
3. 在切面类中,需要使用 @Aspect 和 @Component 注解进行注解。
4. 在 Java 配置类中,需要使用 @Configuration 和 @EnableAspectJAutoProxy 注解进行注解。
5. 在测试用主类中,需要加载 Java 配置类,并通过 context.getBean() 方法获取 Bean 实例。
以上是我提供的代码示例,希望能够对您有所帮助。
阅读全文
相关推荐

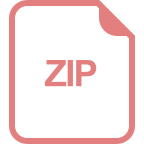
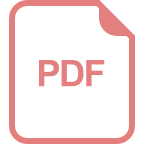
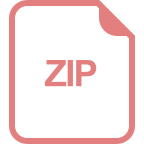
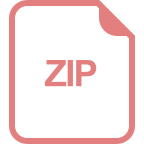
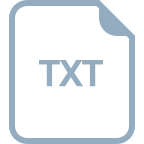
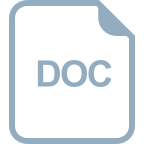
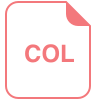
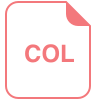
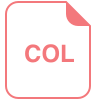
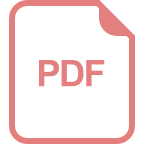
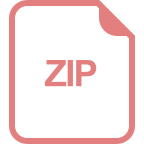
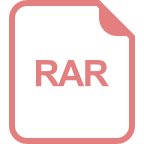
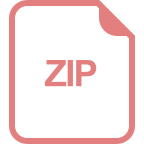
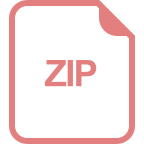
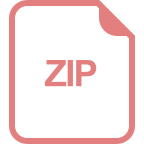
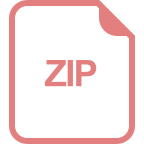