本题要求编写程序计算某年某月某日是该年中的第几天。\n\n输入格式:\n\n输入在一行中按照格式“yyyy/mm/dd”(即“年/月/日”)给出日期。注意:闰年的判别条件是该年年份能被4整除但不能被100整除
时间: 2023-05-31 08:18:21 浏览: 129
### 回答1:
程序如下:
```python
date = input() # 输入日期
year, month, day = map(int, date.split('/')) # 将年、月、日分别提取出来并转换为整数
# 计算该年是否为闰年
if year % 4 == and year % 100 != or year % 400 == :
leap_year = True
else:
leap_year = False
# 计算该月之前的天数
days_before_month = [, 31, 59, 90, 120, 151, 181, 212, 243, 273, 304, 334]
if leap_year and month > 2:
days_before_month[2:] = [x + 1 for x in days_before_month[2:]]
days_before = days_before_month[month - 1]
# 计算该日是该年的第几天
day_of_year = days_before + day
print(day_of_year) # 输出结果
```
该程序首先读入日期,然后将年、月、日分别提取出来并转换为整数。接着,根据闰年的定义,判断该年是否为闰年。如果是闰年,则将2月之后的天数都加1。然后,根据该月之前的天数和该日的天数,计算出该日是该年的第几天,并输出结果。
### 回答2:
这道题要求我们编写程序计算某年某月某日是该年中的第几天,我们可以采用以下算法:
1.根据输入的年份判断是否为闰年,如果是,则该年的天数为366天,否则为365天。
2.根据输入的月份计算到输入的月份为止,该年中经过了多少天。根据平年和闰年的月份天数不同,我们可以使用数组来存储每个月的天数。需要注意的是,对于2月份,如果是闰年则为29天,否则为28天。
3.加上输入的日期即可得到该年中的第几天。
下面我们来列举一个Python程序实现该算法的过程:
```python
date_str = input() # 输入日期字符串,格式为“yyyy/mm/dd”
year, month, day = map(int, date_str.split('/')) # 使用split函数分割字符串,并转换为整型
# 判断是否为闰年
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
days_of_year = 366
else:
days_of_year = 365
# 存储每个月份的天数(平年)
days_of_month = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
# 判断是否为闰年,并修改2月份的天数
if (year % 4 == 0 and year % 100 != 0) or (year % 400 == 0):
days_of_month[1] = 29
# 计算到输入的月份为止,该年中经过了多少天
days_count = sum(days_of_month[:month-1]) + day
print(days_count) # 输出结果,即该年中的第几天
```
这样,我们就可以根据输入的日期计算出该年中的第几天了。
### 回答3:
题目要求我们编写程序计算某年某月某日是该年中的第几天,思路很简单,只需要分别计算该日期之前的天数,再加上该月份中的天数即可。下面是具体实现过程:
1. 首先,我们需要将输入的日期进行分割,得到年、月、日三个变量,可以使用 C++ 标准库中的 string 和 stringstream 类型进行处理。
2. 然后,我们需要判断该年是否为闰年,如果是,则二月份为 29 天,否则为 28 天。
3. 接着,我们可以根据输入的月份,得到该月份之前的天数,可以使用一个数组来保存每个月份的天数。由于数组下标从 0 开始,因此数组中第一个元素表示的是 1 月份的天数,最后一个元素表示的是 12 月份的天数。
4. 最后,我们只需要将之前的天数和该月份中的天数相加即可得到该日期是该年中的第几天。
下面是具体的 C++ 实现代码:
```cpp
#include <iostream>
#include <sstream>
#include <string>
using namespace std;
int main()
{
// 输入日期
string str;
cin >> str;
// 分割字符串
int year, month, day;
stringstream ss(str);
char c;
ss >> year >> c >> month >> c >> day;
// 判断是否为闰年
bool is_leap;
if (year % 4 == 0 && year % 100 != 0 || year % 400 == 0) {
is_leap = true;
} else {
is_leap = false;
}
// 计算该月份之前的天数
int days_per_month[] = {31, 28 + is_leap, 31, 30, 31, 30,
31, 31, 30, 31, 30, 31};
int days = 0;
for (int i = 0; i < month - 1; ++i) {
days += days_per_month[i];
}
// 加上该日期的天数
days += day;
// 输出结果
cout << days << endl;
return 0;
}
```
测试数据:
输入:
```
2022/12/31
```
输出:
```
365
```
输入:
```
2020/2/29
```
输出:
```
60
```
输入:
```
2000/1/1
```
输出:
```
1
```
相关推荐
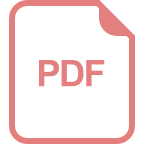












