帮我用python写一段可以定时发送微信消息的代码
时间: 2024-02-15 22:03:02 浏览: 191
你可以使用itchat库来实现定时发送微信消息的功能。以下是一个示例代码,可以每隔一分钟发送一条消息给指定的微信好友:
```python
import itchat
import time
# 登录微信
itchat.auto_login()
# 微信好友的昵称(需要先添加好友)
friend_name = '好友的昵称'
# 定时发送消息
while True:
# 获取当前时间
current_time = time.strftime('%H:%M', time.localtime())
# 判断是否到达指定时间
if current_time == '09:00':
# 发送消息
itchat.send('早上好!', toUserName=friend_name)
# 休眠1分钟
time.sleep(60)
```
需要注意的是,需要先添加好友并获取好友的昵称,才能发送消息。另外,要保持电脑处于开机状态,才能让程序一直运行。
相关问题
帮我用python写一段自动定时发送微信消息的代码
好的,使用Python可以使用itchat库来实现自动发送微信消息。以下是一个简单的示例代码,可以帮助你自动发送微信消息:
```python
import itchat
import time
# 登录微信
itchat.auto_login(hotReload=True)
# 自动发送消息
def send_msg_to_friend(friend_name, msg_content, send_time):
# 获取好友列表
friend_list = itchat.get_friends(update=True)
# 根据好友名称查找好友
for friend in friend_list:
if friend['RemarkName'] == friend_name:
# 发送消息
itchat.send(msg_content, toUserName=friend['UserName'])
print('已发送消息:', msg_content)
break
# 定时发送消息
while True:
now_time = time.strftime('%H:%M:%S', time.localtime())
if now_time == send_time:
itchat.send(msg_content, toUserName=friend['UserName'])
print('已发送消息:', msg_content)
break
time.sleep(1)
if __name__ == '__main__':
# 好友名称
friend_name = '好友的备注名'
# 要发送的消息内容
msg_content = '你好,这是一条自动发送的消息。'
# 发送时间,格式为'时:分:秒'
send_time = '10:00:00'
# 发送消息
send_msg_to_friend(friend_name, msg_content, send_time)
```
注意:在使用该代码之前,你需要先使用itchat库登录微信,并且需要将好友的备注名替换为你要发送消息的好友的备注名。同时,你需要将发送时间设置为你想要发送消息的时间。
python微信群定时发送消息_Python3 itchat实现微信定时发送群消息的实例代码
好的,下面是实现微信群定时发送消息的 Python3 itchat 实例代码:
```python
import itchat
import time
from apscheduler.schedulers.background import BackgroundScheduler
# 登录微信
itchat.auto_login(hotReload=True)
# 获取群聊对象
group = itchat.search_chatrooms(name='群聊名称')[0]
# 定义定时发送消息的函数
def send_msg():
group.send('定时发送的消息')
# 创建定时任务
scheduler = BackgroundScheduler()
scheduler.add_job(send_msg, 'interval', minutes=1) # 每隔1分钟发送一次消息
scheduler.start()
# 让程序一直运行
while True:
time.sleep(10)
```
需要注意的是,这段代码需要安装 `itchat` 和 `apscheduler` 两个库,可以使用以下命令进行安装:
```
pip install itchat
pip install apscheduler
```
另外,代码中的 `群聊名称` 需要替换成你要发送消息的群聊的名称。
阅读全文
相关推荐
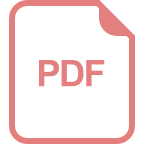
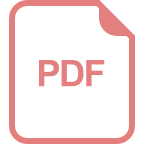
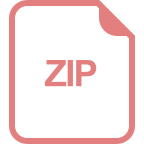
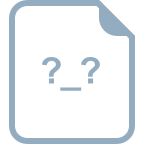
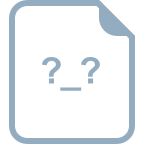
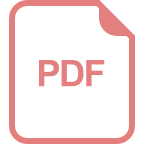
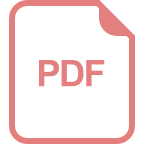
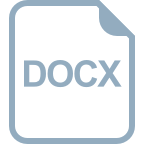
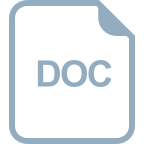
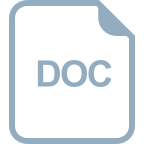
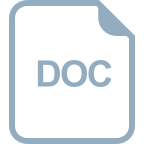


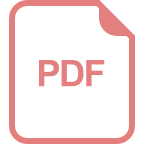