keras的自定义函数保存在哪里
时间: 2023-12-20 14:05:33 浏览: 26
Keras的自定义函数可以保存在Python文件中,然后通过`from xxx import yyy`的方式进行引用。如果你创建了一个自定义的层或模型,可以将其保存在一个Python文件中,例如:
```python
# 自定义的层
from keras.layers import Layer
class MyLayer(Layer):
def __init__(self, output_dim, **kwargs):
self.output_dim = output_dim
super(MyLayer, self).__init__(**kwargs)
def build(self, input_shape):
self.kernel = self.add_weight(name='kernel',
shape=(input_shape[1], self.output_dim),
initializer='uniform',
trainable=True)
super(MyLayer, self).build(input_shape)
def call(self, x):
return K.dot(x, self.kernel)
def compute_output_shape(self, input_shape):
return (input_shape[0], self.output_dim)
# 自定义的模型
from keras.models import Model
from keras.layers import Input, Dense
def MyModel(input_shape, output_shape):
inputs = Input(shape=input_shape)
x = Dense(32, activation='relu')(inputs)
x = MyLayer(output_shape)(x)
model = Model(inputs=inputs, outputs=x)
return model
```
然后在训练模型或者使用该层时,可以通过以下方式进行引用:
```python
from my_module import MyLayer, MyModel
model = MyModel(input_shape=(10,), output_shape=5)
model.compile(loss='mse', optimizer='adam')
model.fit(x_train, y_train, epochs=10)
layer = MyLayer(output_dim=10)
```
需要注意的是,如果你的自定义函数保存在一个Python文件中,需要确保该文件所在的目录在Python解释器的搜索路径中,或者通过`sys.path.append()`将该目录添加到搜索路径中。
相关推荐
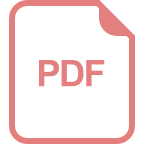
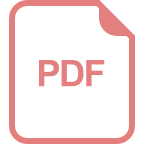














