cjson解析下面的数据
时间: 2024-03-17 14:43:18 浏览: 18
假设需要解析的JSON数据如下:
```
{
"name": "John",
"age": 30,
"city": "New York",
"hobbies": ["reading", "traveling", "swimming"],
"education": {
"degree": "Master",
"major": "Computer Science"
}
}
```
使用cJSON库进行解析的示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include "cJSON.h"
int main() {
char *json_str = "{\"name\":\"John\",\"age\":30,\"city\":\"New York\",\"hobbies\":[\"reading\",\"traveling\",\"swimming\"],\"education\":{\"degree\":\"Master\",\"major\":\"Computer Science\"}}";
cJSON *root = cJSON_Parse(json_str);
if (root == NULL) {
printf("Error before: [%s]\n", cJSON_GetErrorPtr());
return 1;
}
cJSON *name = cJSON_GetObjectItemCaseSensitive(root, "name");
if (cJSON_IsString(name) && (name->valuestring != NULL)) {
printf("Name: %s\n", name->valuestring);
}
cJSON *age = cJSON_GetObjectItemCaseSensitive(root, "age");
if (cJSON_IsNumber(age)) {
printf("Age: %d\n", age->valueint);
}
cJSON *city = cJSON_GetObjectItemCaseSensitive(root, "city");
if (cJSON_IsString(city) && (city->valuestring != NULL)) {
printf("City: %s\n", city->valuestring);
}
cJSON *hobbies = cJSON_GetObjectItemCaseSensitive(root, "hobbies");
if (cJSON_IsArray(hobbies)) {
int i, size = cJSON_GetArraySize(hobbies);
printf("Hobbies:\n");
for (i = 0; i < size; i++) {
cJSON *item = cJSON_GetArrayItem(hobbies, i);
if (cJSON_IsString(item) && (item->valuestring != NULL)) {
printf(" %s\n", item->valuestring);
}
}
}
cJSON *education = cJSON_GetObjectItemCaseSensitive(root, "education");
if (cJSON_IsObject(education)) {
cJSON *degree = cJSON_GetObjectItemCaseSensitive(education, "degree");
if (cJSON_IsString(degree) && (degree->valuestring != NULL)) {
printf("Education - Degree: %s\n", degree->valuestring);
}
cJSON *major = cJSON_GetObjectItemCaseSensitive(education, "major");
if (cJSON_IsString(major) && (major->valuestring != NULL)) {
printf("Education - Major: %s\n", major->valuestring);
}
}
cJSON_Delete(root);
return 0;
}
```
运行结果:
```
Name: John
Age: 30
City: New York
Hobbies:
reading
traveling
swimming
Education - Degree: Master
Education - Major: Computer Science
```
解析过程中,先使用cJSON_Parse函数将JSON字符串解析成一个cJSON对象(即root)。然后,使用cJSON_GetObjectItemCaseSensitive函数分别获取其中的各个成员对象,并使用不同的cJSON类型判断函数(如cJSON_IsString、cJSON_IsNumber、cJSON_IsArray等)判断其类型。最后,使用各自的value获取成员对象的值。最后,使用cJSON_Delete函数释放cJSON对象。
相关推荐
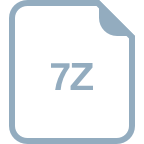














