用c语言写按位序插入单链表的函数
时间: 2024-05-03 07:22:05 浏览: 18
好的,我可以帮您解答这个问题。以下是用 C 语言写按位序插入单链表的函数的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int data;
struct node *next;
} Node;
void InsertNode(Node **head, int data, int position) {
Node *newNode, *tempNode;
int i;
newNode = (Node *)malloc(sizeof(Node));
if (newNode == NULL) {
printf("Memory allocation failed\n");
exit(0);
}
newNode->data = data;
if (position == 1 || *head == NULL) {
newNode->next = *head;
*head = newNode;
} else {
tempNode = *head;
for (i = 1; i < position - 1 && tempNode != NULL; i++) {
tempNode = tempNode->next;
}
if (tempNode == NULL) {
printf("Position is out of range\n");
free(newNode);
return;
}
newNode->next = tempNode->next;
tempNode->next = newNode;
}
}
void TraverseList(Node *head) {
Node *tempNode;
tempNode = head;
while (tempNode != NULL) {
printf("%d -> ", tempNode->data);
tempNode = tempNode->next;
}
printf("NULL\n");
}
int main() {
Node *head = NULL;
InsertNode(&head, 10, 1);
InsertNode(&head, 20, 2);
InsertNode(&head, 30, 1);
InsertNode(&head, 40, 4);
InsertNode(&head, 50, 3);
TraverseList(head);
return 0;
}
```
该程序在单链表的第 `position` 个位置插入一个数据为 `data` 的节点。如果 `position` 是 1,新节点将成为新的头节点;如果单链表为空,则新节点将成为新的头节点。如果 `position` 超出了单链表的长度,则将在末尾插入新节点。
相关推荐
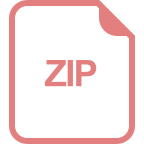














