怎么在拍照时获取该图片的uri
时间: 2024-02-15 22:05:27 浏览: 87
在拍照时获取图片的uri,可以通过以下步骤实现:
1. 在AndroidManifest.xml文件中添加文件提供器(FileProvider):
```xml
<provider
android:name="androidx.core.content.FileProvider"
android:authorities="${applicationId}.fileProvider"
android:exported="false"
android:grantUriPermissions="true">
<meta-data
android:name="android.support.FILE_PROVIDER_PATHS"
android:resource="@xml/file_paths" />
</provider>
```
2. 在res/xml文件夹中创建一个名为file_paths.xml的文件,并在其中指定保存图片的路径:
```xml
<paths xmlns:android="http://schemas.android.com/apk/res/android">
<external-files-path
name="my_images"
path="Pictures" />
</paths>
```
3. 在拍照的Activity中,创建一个文件对象并将其保存在指定的路径下:
```java
File photoFile = null;
try {
photoFile = File.createTempFile(
"IMG_", /* prefix */
".jpg", /* suffix */
getExternalFilesDir(Environment.DIRECTORY_PICTURES) /* directory */
);
} catch (IOException ex) {
// Error occurred while creating the File
...
}
// Save a file: path for use with ACTION_VIEW intents
String currentPhotoPath = photoFile.getAbsolutePath();
```
4. 启动相机应用,并在onActivityResult()方法中获取拍摄后的照片uri:
```java
private static final int REQUEST_IMAGE_CAPTURE = 1;
private void dispatchTakePictureIntent() {
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// Ensure that there's a camera activity to handle the intent
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
// Create the File where the photo should go
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
...
}
// Continue only if the File was successfully created
if (photoFile != null) {
Uri photoURI = FileProvider.getUriForFile(this,
BuildConfig.APPLICATION_ID + ".fileProvider",
photoFile);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE);
}
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
// Get the uri of the captured image
Uri uri = Uri.fromFile(photoFile);
...
}
}
```
注意:在Android 10及以上版本中,需要使用FileProvider来获取文件uri,而不是直接使用file://协议。
阅读全文
相关推荐
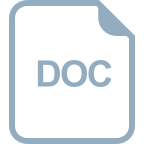
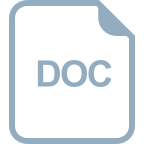
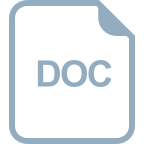
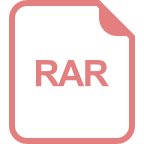
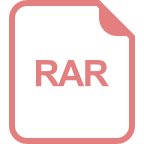
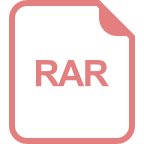
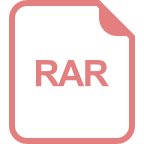
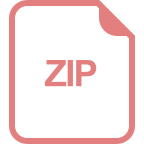
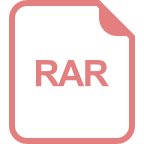
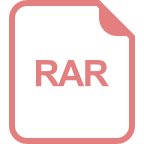
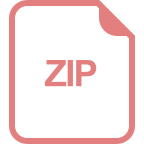
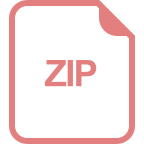
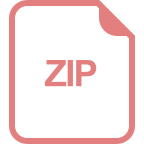
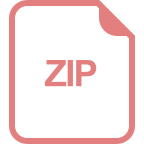
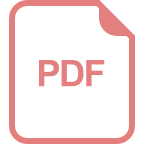


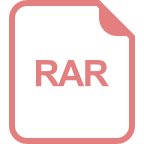
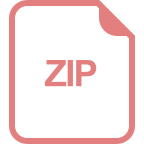