• Design a Stack class (Eg 8 9 w) When the element pushed into the stack exceeds the capacity of the stack, it will throw a stack-full exception; When pop an element out of the stack when the stack is full, it will throw a stack-empty exception.
时间: 2024-03-01 14:54:18 浏览: 16
Sure, I can help you design a Stack class with the mentioned requirements. Here is a simple implementation of the Stack class in Python:
```
class Stack:
def __init__(self, capacity):
self.capacity = capacity
self.stack = []
def push(self, element):
if len(self.stack) == self.capacity:
raise Exception("Stack is full")
self.stack.append(element)
def pop(self):
if len(self.stack) == 0:
raise Exception("Stack is empty")
return self.stack.pop()
def is_empty(self):
return len(self.stack) == 0
def is_full(self):
return len(self.stack) == self.capacity
```
In the constructor, we set the capacity of the stack and initialize an empty list to store the elements. The `push` method adds an element to the stack but raises an exception if the stack is full. The `pop` method removes and returns the top element from the stack but raises an exception if the stack is empty. The `is_empty` and `is_full` methods return True or False depending on whether the stack is empty or full.
相关推荐
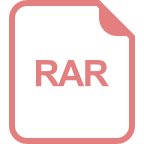
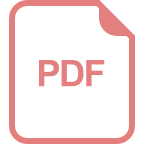














