代码优化 public String getApplyId() { ValueOperations<String,Long> value = redisTemplate.opsForValue(); LocalDateTime dateTime = LocalDateTime.now(); String date = dateTime.format(DateTimeFormatter.ofPattern("yyMMdd")); Long count = 0L; String append = "Reconciliation:AD" + date; String dateString = "AD" + date; if (value.get(append) == null) { // 获取数据库的值 LambdaQueryWrapper<ReconciliationApply> wrapper = new LambdaQueryWrapper<>(); wrapper.eq(ReconciliationApply::getPurchaseCode, dateString); ReconciliationApply selectOne = applyMapper.selectOne(wrapper); if (selectOne == null) { value.setIfAbsent(append, count); redisTemplate.expire(append, 1, TimeUnit.DAYS); } else { String purchaseCode = selectOne.getPurchaseCode(); String substring = purchaseCode.substring(8, 13); value.setIfAbsent(append, Long.parseLong(substring)); redisTemplate.expire(append, 1, TimeUnit.DAYS); } } Long increment = value.increment(append, 1); String str = String.valueOf(increment); return getSequence(dateString, str); } /** * 格式化 key * * @param str * @return */ public static String getSequence(String dateString, String str) { StringBuilder stringJoiner = new StringBuilder(dateString); int len = str.length(); // 取决于业务规模 4 if (len == DEFAULT_LENGTH) { return stringJoiner.append(str).toString(); } if (str.length() > DEFAULT_LENGTH) { throw new RuntimeException("申请单号已使用完成,请明天再申请"); } int rest = DEFAULT_LENGTH - len; for (int i = 0; i < rest; i++) { stringJoiner.append("0"); } stringJoiner.append(str); return stringJoiner.toString(); }
时间: 2023-12-02 20:06:22 浏览: 68
可以考虑对代码进行如下优化:
1. 将 Redis 的操作放到单独的方法中,方便复用和维护。
2. 对于 Redis 中的值的获取和设置,可以使用 Redis 的 get/set 方法代替 opsForValue() 方法,简化代码。
3. 对于 Redis 中的键的格式,可以使用 String.format() 方法代替 StringBuilder,使代码更加简洁。
4. 对于日期和时间的格式化,可以使用 Java 8 中的 DateTimeFormatter 做进一步优化。
综上所述,代码可以优化为:
```
public String getApplyId() {
LocalDateTime dateTime = LocalDateTime.now();
String dateString = "AD" + dateTime.format(DateTimeFormatter.ofPattern("yyMMdd"));
String key = String.format("Reconciliation:%s", dateString);
ValueOperations<String, String> valueOps = redisTemplate.opsForValue();
String value = valueOps.get(key);
if (value == null) {
ReconciliationApply apply = applyMapper.selectOne(
new LambdaQueryWrapper<ReconciliationApply>().eq(ReconciliationApply::getPurchaseCode, dateString));
if (apply == null) {
valueOps.setIfAbsent(key, "0001");
} else {
String purchaseCode = apply.getPurchaseCode();
String suffix = purchaseCode.substring(8);
valueOps.setIfAbsent(key, suffix);
}
redisTemplate.expire(key, 1, TimeUnit.DAYS);
}
long sequence = valueOps.increment(key, 1);
String str = String.format("%04d", sequence);
return dateString + str;
}
```
阅读全文
相关推荐

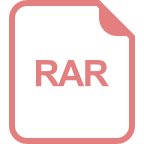
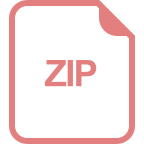
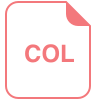
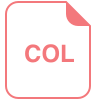
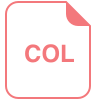
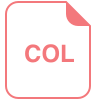
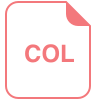
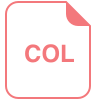
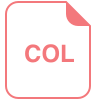
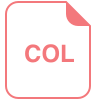



