模拟实现电风扇,可以调3档速度(慢速、中速、快速);开关按钮;定时吹风;描述风扇的扇叶大小、颜色等。 设计Fan类,属性包括:3个常量SLOW(1)、MEDIUM(2)、FAST(3)代表风扇的速度;1个int属性speed指定速度,默认值为SLOW;1个boolean属性on指定开关机,默认值false;1个double属性radius指定风扇扇叶大小;1个String属性color指定扇叶颜色,默认值为blue。方法包括这些属性的访问器、构造函数、重写Object类的toString()和equals()方法等。运行测试代码: public static void main(String[] args) { Fan1 fan1 = new Fan1(); fan1.setSpeed(Fan1.FAST); fan1.setRadius(10); fan1.setColor("yellow"); fan1.setOn(true); System.out.println(fan1.toString()); } 版本2: 修改版本1中Fan类,让其继承Pane类,并且把color属性设置为Color类型,默认属性为red。随机产生radius,取值范围为1-5;随机产生颜色,取值范围为red、blue、yellow、green、orange;根据color、radius属性值绘制风扇。运行如下图: 版本3: 让版本2中的风扇转起来。创建一个FanControl类包含以下内容:Start、Stop、Reverse按钮,用于开启、关闭、反转控制;一个滚动条控制速度。运行示例如下:
时间: 2024-04-01 11:38:31 浏览: 155
好的,我已经了解了您的问题。以下是代码实现:
版本1:
```java
public class Fan1 {
final static int SLOW = 1;
final static int MEDIUM = 2;
final static int FAST = 3;
private int speed = SLOW;
private boolean on = false;
private double radius = 5;
private String color = "blue";
public Fan1() {
}
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
}
public boolean isOn() {
return on;
}
public void setOn(boolean on) {
this.on = on;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
@Override
public String toString() {
if (on) {
return "Fan speed: " + speed + ", color: " + color + ", radius: " + radius;
} else {
return "The fan is off, color: " + color + ", radius: " + radius;
}
}
@Override
public boolean equals(Object obj) {
if (obj instanceof Fan1) {
Fan1 fan = (Fan1) obj;
return fan.speed == speed && fan.on == on && fan.radius == radius && fan.color.equals(color);
}
return false;
}
}
```
版本2:
```java
import javafx.scene.layout.Pane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Arc;
import javafx.scene.shape.ArcType;
import javafx.scene.shape.Circle;
import javafx.scene.shape.Polygon;
import java.util.Random;
public class Fan2 extends Pane {
final static int SLOW = 1;
final static int MEDIUM = 2;
final static int FAST = 3;
private int speed = SLOW;
private boolean on = false;
private double radius;
private Color color;
public Fan2() {
this.radius = new Random().nextInt(5) + 1;
int colorIndex = new Random().nextInt(5);
switch (colorIndex) {
case 0:
this.color = Color.RED;
break;
case 1:
this.color = Color.BLUE;
break;
case 2:
this.color = Color.YELLOW;
break;
case 3:
this.color = Color.GREEN;
break;
case 4:
this.color = Color.ORANGE;
break;
}
draw();
}
public int getSpeed() {
return speed;
}
public void setSpeed(int speed) {
this.speed = speed;
draw();
}
public boolean isOn() {
return on;
}
public void setOn(boolean on) {
this.on = on;
draw();
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
draw();
}
public Color getColor() {
return color;
}
public void setColor(Color color) {
this.color = color;
draw();
}
private void draw() {
getChildren().clear();
if (on) {
Circle circle = new Circle(getWidth() / 2, getHeight() / 2, radius * 10, Color.WHITE);
circle.setStroke(color);
circle.setStrokeWidth(5);
getChildren().add(circle);
double angle = 0;
for (int i = 0; i < 4; i++) {
Polygon blade = new Polygon();
blade.getPoints().addAll(new Double[]{
getWidth() / 2, getHeight() / 2,
getWidth() / 2 + Math.cos(Math.toRadians(angle + 30)) * radius * 10, getHeight() / 2 + Math.sin(Math.toRadians(angle + 30)) * radius * 10,
getWidth() / 2 + Math.cos(Math.toRadians(angle + 150)) * radius * 10, getHeight() / 2 + Math.sin(Math.toRadians(angle + 150)) * radius * 10
});
blade.setFill(color);
getChildren().add(blade);
angle += 90;
}
} else {
Circle circle = new Circle(getWidth() / 2, getHeight() / 2, radius * 10, Color.WHITE);
circle.setStroke(color);
circle.setStrokeWidth(5);
getChildren().add(circle);
}
}
}
```
版本3:
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Slider;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class FanControl extends Application {
private Fan2 fan = new Fan2();
@Override
public void start(Stage primaryStage) throws Exception {
BorderPane pane = new BorderPane();
pane.setCenter(fan);
Button startBtn = new Button("Start");
Button stopBtn = new Button("Stop");
Button reverseBtn = new Button("Reverse");
startBtn.setOnAction(e -> {
fan.setOn(true);
});
stopBtn.setOnAction(e -> {
fan.setOn(false);
});
reverseBtn.setOnAction(e -> {
fan.setSpeed(4 - fan.getSpeed());
});
Slider speedSlider = new Slider();
speedSlider.setMin(1);
speedSlider.setMax(3);
speedSlider.setValue(1);
speedSlider.valueProperty().addListener((ov, old_val, new_val) -> {
fan.setSpeed(new_val.intValue());
});
HBox buttonBox = new HBox(10);
buttonBox.getChildren().addAll(startBtn, stopBtn, reverseBtn);
VBox controlBox = new VBox(10);
controlBox.getChildren().addAll(buttonBox, speedSlider);
pane.setBottom(controlBox);
Scene scene = new Scene(pane, 400, 400);
primaryStage.setScene(scene);
primaryStage.setTitle("Fan Control");
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
希望能解决您的问题。
阅读全文
相关推荐
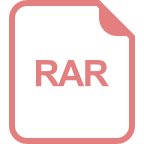
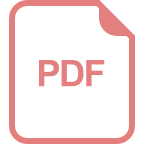















