就是你刚刚说的那个使用QFile和QDataStream类来结合文件映射进行编码bmp图的功能封装成函数
时间: 2024-05-10 12:21:25 浏览: 153
以下是一个使用QFile和QDataStream类来结合文件映射进行编码bmp图的功能封装成函数的示例:
```cpp
#include <QFile>
#include <QDataStream>
bool encodeBmpFile(const QString& fileName, const QImage& image)
{
QFile file(fileName);
if (!file.open(QIODevice::WriteOnly))
return false;
int width = image.width();
int height = image.height();
int bitsPerPixel = image.depth();
int bytesPerLine = (width * bitsPerPixel + 31) / 32 * 4;
int dataSize = bytesPerLine * height;
int fileSize = dataSize + 54;
QByteArray data;
data.resize(fileSize);
// BMP header
data[0] = 'B';
data[1] = 'M';
data[2] = fileSize & 0xff;
data[3] = (fileSize >> 8) & 0xff;
data[4] = (fileSize >> 16) & 0xff;
data[5] = (fileSize >> 24) & 0xff;
data[10] = 54;
data[14] = 40;
data[18] = width & 0xff;
data[19] = (width >> 8) & 0xff;
data[20] = (width >> 16) & 0xff;
data[21] = (width >> 24) & 0xff;
data[22] = height & 0xff;
data[23] = (height >> 8) & 0xff;
data[24] = (height >> 16) & 0xff;
data[25] = (height >> 24) & 0xff;
data[26] = 1;
data[28] = bitsPerPixel;
// BMP data
uchar* dest = reinterpret_cast<uchar*>(data.data() + 54);
const uchar* src = image.constBits();
for (int y = 0; y < height; ++y) {
memcpy(dest, src, bytesPerLine);
dest += bytesPerLine;
src += image.bytesPerLine();
}
// Write data to file
QDataStream stream(&file);
stream.writeRawData(data.constData(), fileSize);
return true;
}
```
该函数接受两个参数:要写入的文件名和要编码的图像对象。函数首先打开文件并计算文件大小,然后创建一个字节数组来存储BMP文件头和图像数据。接下来,该函数设置BMP文件头和将图像数据复制到字节数组中。最后,将数据写入文件并返回成功标志。
阅读全文
相关推荐
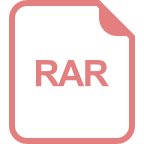



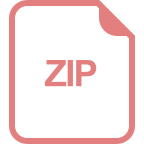
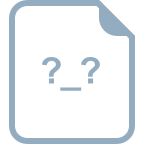
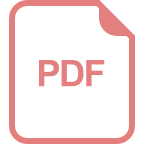









