写一个方法:function2,参数为:文件路径名。 功能要求: ① 获取该路径下所有 Excel 文件(含子文件夹下),并把 Excel 文件最后修改时间为:一 周之内的 Excel 内容,合并到 mzy-merge.xlsx 文件中。 ② mzy-merge.xlsx 文件中,每个被合并文件内容最前部插入:该文件绝对路径,且背景色 为黄色,字体为红色。 ③ 返回值为:mzy-merge.xlsx 文件的绝对路径字符串
时间: 2024-01-21 20:17:00 浏览: 13
以下是一个实现上述功能的方法:
```javascript
const fs = require('fs');
const path = require('path');
const xlsx = require('xlsx');
function function2(dirPath) {
const mergeFilename = 'mzy-merge.xlsx';
const mergeFilePath = path.join(dirPath, mergeFilename);
// 如果 mzy-merge.xlsx 文件不存在,则新建一个
if (!fs.existsSync(mergeFilePath)) {
const wb = xlsx.utils.book_new();
xlsx.writeFile(wb, mergeFilePath);
}
// 获取一周之内修改过的 Excel 文件
const oneWeekAgo = Date.now() - 7 * 24 * 60 * 60 * 1000;
const files = getAllFiles(dirPath).filter(filePath => {
if (!filePath.endsWith('.xlsx')) {
return false;
}
const stats = fs.statSync(filePath);
return stats.isFile() && stats.mtimeMs >= oneWeekAgo;
});
// 合并 Excel 文件内容到 mzy-merge.xlsx 文件
const wb = xlsx.readFile(mergeFilePath);
const ws = wb.Sheets['Sheet1'];
files.forEach(filePath => {
const fileContent = xlsx.readFile(filePath);
const sheetNameList = fileContent.SheetNames;
sheetNameList.forEach(sheetName => {
const sheetContent = fileContent.Sheets[sheetName];
const cellValue = sheetContent['!ref'] ? sheetContent['!ref'] : 'A1';
const newCell = {
t: 's',
v: filePath,
s: {
fill: {
fgColor: { rgb: 'FFFF00' }
},
font: {
color: { rgb: 'FF0000' }
}
}
};
xlsx.utils.sheet_add_aoa(sheetContent, [[], [], [], [], []], {
origin: 'A1'
});
sheetContent['A1'] = newCell;
xlsx.utils.book_append_sheet(wb, sheetContent, sheetName);
});
});
xlsx.writeFile(wb, mergeFilePath);
return mergeFilePath;
}
// 获取指定目录下的所有文件(包括子目录下的文件)
function getAllFiles(dirPath) {
const files = [];
const dirs = [dirPath];
while (dirs.length > 0) {
const currentDir = dirs.shift();
const currentFiles = fs.readdirSync(currentDir);
currentFiles.forEach(filename => {
const filePath = path.join(currentDir, filename);
const stats = fs.statSync(filePath);
if (stats.isDirectory()) {
dirs.push(filePath);
} else {
files.push(filePath);
}
});
}
return files;
}
```
调用示例:
```javascript
const mergeFilePath = function2('/path/to/directory');
console.log(mergeFilePath);
```
相关推荐
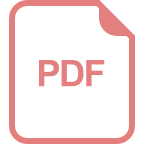
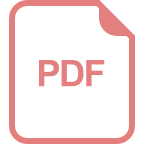
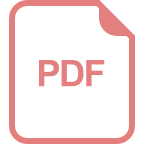









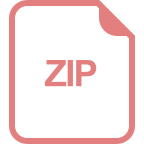
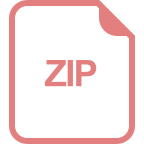
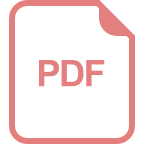
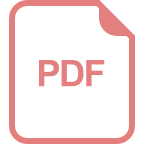
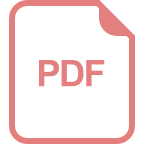
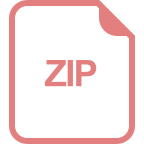