js制作图书馆管理系统代码实现
时间: 2023-08-25 11:04:36 浏览: 123
由于图书馆管理系统的代码实现较为复杂,包括前端页面、后端程序以及数据库的设计和操作,因此在这里无法给出完整的代码实现。不过我可以给你提供一个基本的框架,供你参考:
1. 前端页面:
- 登录页面:实现用户登录功能,验证用户输入的用户名和密码是否正确。
- 主页面:显示图书馆的基本信息,包括图书馆的名称、地址、联系方式等。
- 图书查询页面:允许用户查询图书信息,包括书名、作者、出版社等。
- 借阅记录页面:显示读者的借阅记录,包括借阅时间、归还时间、借阅状态等。
- 读者信息管理页面:允许管理员管理读者信息,包括添加读者、删除读者、修改读者信息等。
- 图书信息管理页面:允许管理员管理图书信息,包括添加图书、删除图书、修改图书信息等。
2. 后端程序:
- 实现用户登录和验证功能。
- 实现图书查询和借阅记录查询功能。
- 实现读者信息管理和图书信息管理功能。
- 实现借还书的功能,包括更新借阅记录和图书库存信息。
3. 数据库设计:
- 设计书籍信息、读者信息、借书记录等表格。
- 设计表格之间的关系,如读者信息与借书记录的关系、书籍信息与借书记录的关系等。
- 设计表格的字段,包括书名、作者、出版社、借阅时间、归还时间等。
这是一个基本的框架,具体的代码实现需要根据实际情况进行调整和修改。如果你需要更详细的信息,可以参考一些相关的教程或者书籍。
相关问题
jQuery制作图书管理系统
以下是使用jQuery制作图书管理系统的一些步骤和方法:
1. 首先,需要使用HTML和CSS创建一个基本的页面布局,包括导航栏、表格、表单等元素。
2. 然后,使用jQuery编写JavaScript代码,实现以下功能:
- 从数据库中获取图书信息,并将其显示在表格中。
- 实现图书的增删改查功能,包括添加新书、删除书籍、修改书籍信息和查询书籍。
- 实现图书的借出和归还功能,包括将借阅信息保存到数据库中。
- 实现读者信息的管理,包括添加新读者、删除读者、修改读者信息和查询读者。
3. 在JavaScript代码中,使用Ajax技术与后端服务器进行通信,从而实现数据的传输和存储。
4. 最后,将代码部署到Tomcat服务器上,并使用SQLyog等工具导入和存储数据。
下面是一个简单的示例代码,用于从数据库中获取图书信息并将其显示在表格中:
```javascript
$(document).ready(function() {
$.ajax({
url: "get_books.php", // 后端服务器地址
type: "GET",
dataType: "json",
success: function(data) {
// 将获取到的数据显示在表格中
$.each(data, function(index, book) {
var row = "<tr><td>" + book.title + "</td><td>" + book.author + "</td><td>" + book.publisher + "</td></tr>";
$("#book-table").append(row);
});
},
error: function(jqXHR, textStatus, errorThrown) {
console.log("Error: " + textStatus + " " + errorThrown);
}
});
});
```
nodejs制作图书管理系统
Node.js是一个基于Chrome V8引擎的JavaScript运行环境,可以用于开发服务器端应用程序。下面是使用Node.js制作图书管理系统的步骤:
1.安装Node.js和MySQL数据库,并创建一个名为bookstore的数据库。
2.使用npm安装Express框架和mysql模块,用于搭建服务器和连接数据库。
3.创建一个名为index.js的主入口文件,用于路由分配和启动服务器。
4.创建一个名为router.js的路由文件,用于处理不同的URL请求。
5.创建一个名为db.js的数据库连接文件,用于连接MySQL数据库。
6.创建一个名为book.js的业务逻辑文件,用于实现图书信息的增删改查功能。
7.创建一个名为book.html的前端页面文件,用于展示图书信息和与后端进行交互。
8.在index.js中引入路由文件、数据库连接文件和业务逻辑文件,并启动服务器。
9.在router.js中编写不同URL请求的处理函数,例如获取所有图书信息、添加图书信息、修改图书信息和删除图书信息等。
10.在db.js中编写连接MySQL数据库的代码,并导出连接对象。
11.在book.js中编写业务逻辑代码,例如查询所有图书信息、添加图书信息、修改图书信息和删除图书信息等。
12.在book.html中编写前端页面代码,例如展示所有图书信息、添加图书信息、修改图书信息和删除图书信息等。
下面是一个简单的示例代码:
```javascript
// index.js
const express = require('express');
const router = require('./router');
const db = require('./db');
const book = require('./book');
const app = express();
const port = 3000;
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
router(app, book);
db.connect(() => {
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
});
// router.js
module.exports = (app, book) => {
app.get('/books', book.getAll);
app.post('/books', book.add);
app.put('/books/:id', book.update);
app.delete('/books/:id', book.remove);
};
// db.js
const mysql = require('mysql');
const connection = mysql.createConnection({
host: 'localhost',
user: 'root',
password: 'password',
database: 'bookstore',
});
module.exports = {
connect: (callback) => {
connection.connect((err) => {
if (err) {
console.error(`Error connecting to database: ${err.stack}`);
return; }
console.log(`Connected to database as id ${connection.threadId}`);
callback();
});
},
query: (sql, params, callback) => {
connection.query(sql, params, (err, results, fields) => {
if (err) {
console.error(`Error executing query: ${err.stack}`);
return;
}
callback(results);
});
},
};
// book.js
const db = require('./db');
module.exports = {
getAll: (req, res) => {
db.query('SELECT * FROM books', [], (results) => {
res.json(results);
});
},
add: (req, res) => {
const { title, author, price } = req.body;
db.query('INSERT INTO books (title, author, price) VALUES (?, ?, ?)', [title, author, price], (results) => {
res.json({ message: 'Book added successfully' });
});
},
update: (req, res) => {
const { id } = req.params;
const { title, author, price } = req.body;
db.query('UPDATE books SET title = ?, author = ?, price = ? WHERE id = ?', [title, author, price, id], (results) => {
res.json({ message: 'Book updated successfully' });
});
},
remove: (req, res) => {
const { id } = req.params;
db.query('DELETE FROM books WHERE id = ?', [id], (results) => {
res.json({ message: 'Book deleted successfully' });
});
},
};
// book.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Bookstore</title>
</head>
<body>
<h1>Bookstore</h1>
<table>
<thead>
<tr>
<th>Title</th>
<th>Author</th>
<th>Price</th>
<th>Action</th>
</tr>
</thead>
<tbody id="book-list">
</tbody>
</table>
<form id="add-book-form">
<h2>Add Book</h2>
<div>
<label>Title:</label>
<input type="text" name="title">
</div>
<div>
<label>Author:</label>
<input type="text" name="author">
</div>
<div>
<label>Price:</label>
<input type="number" name="price">
</div>
<button type="submit">Add</button>
</form>
<script>
const bookList = document.getElementById('book-list');
const addBookForm = document.getElementById('add-book-form');
function renderBooks() {
fetch('/books')
.then(response => response.json())
.then(books => {
bookList.innerHTML = '';
books.forEach(book => {
const tr = document.createElement('tr');
const titleTd = document.createElement('td');
const authorTd = document.createElement('td');
const priceTd = document.createElement('td');
const actionTd = document.createElement('td');
const editButton = document.createElement('button');
const deleteButton = document.createElement('button');
titleTd.innerText = book.title;
authorTd.innerText = book.author;
priceTd.innerText = book.price;
editButton.innerText = 'Edit';
deleteButton.innerText = 'Delete';
editButton.addEventListener('click', () => {
const title = prompt('Enter new title:', book.title);
const author = prompt('Enter new author:', book.author);
const price = prompt('Enter new price:', book.price);
fetch(`/books/${book.id}`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ title, author, price })
})
.then(response => response.json())
.then(data => {
alert(data.message);
renderBooks();
});
});
deleteButton.addEventListener('click', () => {
if (confirm('Are you sure you want to delete this book?')) {
fetch(`/books/${book.id}`, {
method: 'DELETE'
})
.then(response => response.json())
.then(data => {
alert(data.message);
renderBooks();
});
}
});
actionTd.appendChild(editButton);
actionTd.appendChild(deleteButton);
tr.appendChild(titleTd);
tr.appendChild(authorTd);
tr.appendChild(priceTd);
tr.appendChild(actionTd);
bookList.appendChild(tr);
});
});
}
addBookForm.addEventListener('submit', (event) => {
event.preventDefault();
const title = addBookForm.elements.title.value;
const author = addBookForm.elements.author.value;
const price = addBookForm.elements.price.value;
fetch('/books', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ title, author, price })
})
.then(response => response.json())
.then(data => {
alert(data.message);
addBookForm.reset();
renderBooks();
});
});
renderBooks();
</script>
</body>
</html>
```
相关推荐
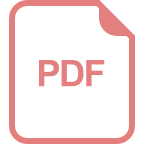
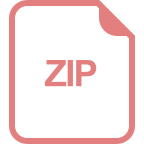
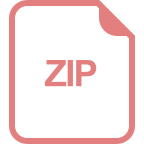









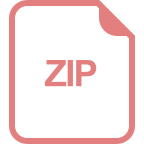
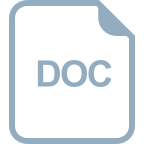
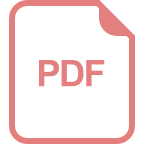
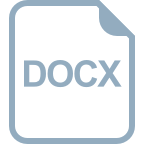