帮我用C语言写一个一元多项式的乘法与加法运算程序
时间: 2023-11-28 11:05:28 浏览: 67
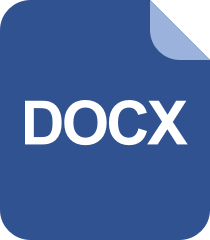
多项式的加法乘法源代码.docx
以下是一元多项式的乘法与加法运算程序的示例代码:
#include <stdio.h>
#include <stdlib.h>
// 定义多项式结构体
typedef struct polynomial {
int coef; // 系数
int exp; // 指数
struct polynomial *next;
} poly;
// 创建多项式
poly *create_poly() {
poly *head = (poly*)malloc(sizeof(poly));
head->next = NULL;
return head;
}
// 插入项
void insert_poly(poly *head, int coef, int exp) {
poly *p = head;
while (p->next != NULL) {
p = p->next;
if (p->exp == exp) { // 同指数项相加
p->coef += coef;
return;
}
}
// 未找到同指数项,插入新项
poly *node = (poly*)malloc(sizeof(poly));
node->coef = coef;
node->exp = exp;
node->next = NULL;
p->next = node;
}
// 打印多项式
void print_poly(poly *head) {
poly *p = head->next;
while (p != NULL) {
printf("%dX^%d", p->coef, p->exp);
if (p->next != NULL && p->next->coef > 0) {
printf("+");
}
p = p->next;
}
printf("\n");
}
// 两个多项式相加
poly *add_poly(poly *p1, poly *p2) {
poly *head = create_poly();
poly *p = head;
while (p1 != NULL && p2 != NULL) {
if (p1->exp > p2->exp) {
insert_poly(head, p1->coef, p1->exp);
p1 = p1->next;
}
else if (p1->exp < p2->exp) {
insert_poly(head, p2->coef, p2->exp);
p2 = p2->next;
}
else {
int coef = p1->coef + p2->coef;
if (coef != 0) {
insert_poly(head, coef, p1->exp);
}
p1 = p1->next;
p2 = p2->next;
}
}
while (p1 != NULL) {
insert_poly(head, p1->coef, p1->exp);
p1 = p1->next;
}
while (p2 != NULL) {
insert_poly(head, p2->coef, p2->exp);
p2 = p2->next;
}
return head;
}
// 两个多项式相乘
poly *mul_poly(poly *p1, poly *p2) {
poly *head = create_poly();
poly *p = head;
while (p1 != NULL) {
poly *q = p2;
while (q != NULL) {
int coef = p1->coef * q->coef;
int exp = p1->exp + q->exp;
insert_poly(head, coef, exp);
q = q->next;
}
p1 = p1->next;
}
return head;
}
int main() {
poly *p1 = create_poly();
poly *p2 = create_poly();
// 插入项
insert_poly(p1, 2, 3);
insert_poly(p1, 3, 2);
insert_poly(p1, 1, 0);
insert_poly(p2, 3, 4);
insert_poly(p2, 1, 2);
insert_poly(p2, -2, 1);
insert_poly(p2, 1, 0);
// 打印原多项式
printf("原多项式1:");
print_poly(p1);
printf("原多项式2:");
print_poly(p2);
// 多项式相加
poly *p3 = add_poly(p1, p2);
printf("相加后多项式:");
print_poly(p3);
// 多项式相乘
poly *p4 = mul_poly(p1, p2);
printf("相乘后多项式:");
print_poly(p4);
return 0;
}
阅读全文
相关推荐
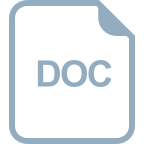
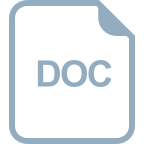



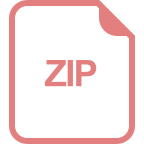



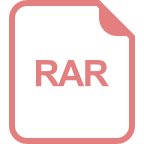
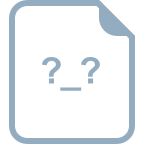
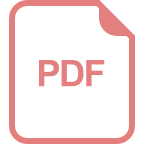
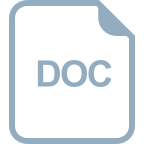
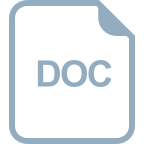



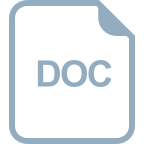