用Java编写学生信息管理系统
时间: 2023-07-18 14:38:35 浏览: 77
好的,学生信息管理系统通常包括以下功能:
1. 学生信息录入:包括学生基本信息、成绩信息等。
2. 学生信息查询:可以按照学生姓名、学号、班级等条件进行查询。
3. 学生成绩统计:可以按照学科、班级等条件进行成绩统计。
4. 学生成绩分析:对学生的成绩进行分析,例如成绩分布情况等。
下面是一个简单的Java实现:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class StudentInfoManagementSystem {
// 定义学生类
static class Student {
String name; // 姓名
String id; // 学号
String className; // 班级
int mathScore; // 数学成绩
int englishScore; // 英语成绩
int chineseScore; // 语文成绩
public Student(String name, String id, String className, int mathScore, int englishScore, int chineseScore) {
this.name = name;
this.id = id;
this.className = className;
this.mathScore = mathScore;
this.englishScore = englishScore;
this.chineseScore = chineseScore;
}
}
// 定义学生列表
static ArrayList<Student> students = new ArrayList<Student>();
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("请选择操作:");
System.out.println("1. 添加学生信息");
System.out.println("2. 查询学生信息");
System.out.println("3. 统计学生成绩");
System.out.println("4. 分析学生成绩");
System.out.println("5. 退出");
int choice = scanner.nextInt();
switch (choice) {
case 1:
addStudent(scanner);
break;
case 2:
queryStudent(scanner);
break;
case 3:
statStudentScore(scanner);
break;
case 4:
analyzeStudentScore();
break;
case 5:
System.exit(0);
break;
default:
System.out.println("无效操作,请重新选择!");
break;
}
}
}
// 添加学生信息
static void addStudent(Scanner scanner) {
System.out.println("请输入学生姓名:");
String name = scanner.next();
System.out.println("请输入学生学号:");
String id = scanner.next();
System.out.println("请输入学生班级:");
String className = scanner.next();
System.out.println("请输入学生数学成绩:");
int mathScore = scanner.nextInt();
System.out.println("请输入学生英语成绩:");
int englishScore = scanner.nextInt();
System.out.println("请输入学生语文成绩:");
int chineseScore = scanner.nextInt();
students.add(new Student(name, id, className, mathScore, englishScore, chineseScore));
System.out.println("添加学生信息成功!");
}
// 查询学生信息
static void queryStudent(Scanner scanner) {
System.out.println("请选择查询方式:");
System.out.println("1. 按姓名查询");
System.out.println("2. 按学号查询");
System.out.println("3. 按班级查询");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入学生姓名:");
String name = scanner.next();
for (Student student : students) {
if (student.name.equals(name)) {
System.out.println(student.id + " " + student.name + " " + student.className + " " +
student.mathScore + " " + student.englishScore + " " + student.chineseScore);
}
}
break;
case 2:
System.out.println("请输入学生学号:");
String id = scanner.next();
for (Student student : students) {
if (student.id.equals(id)) {
System.out.println(student.id + " " + student.name + " " + student.className + " " +
student.mathScore + " " + student.englishScore + " " + student.chineseScore);
}
}
break;
case 3:
System.out.println("请输入学生班级:");
String className = scanner.next();
for (Student student : students) {
if (student.className.equals(className)) {
System.out.println(student.id + " " + student.name + " " + student.className + " " +
student.mathScore + " " + student.englishScore + " " + student.chineseScore);
}
}
break;
default:
System.out.println("无效操作,请重新选择!");
break;
}
}
// 统计学生成绩
static void statStudentScore(Scanner scanner) {
System.out.println("请选择统计方式:");
System.out.println("1. 按班级统计");
System.out.println("2. 按学科统计");
int choice = scanner.nextInt();
switch (choice) {
case 1:
System.out.println("请输入班级名称:");
String className = scanner.next();
int mathTotalScore = 0;
int englishTotalScore = 0;
int chineseTotalScore = 0;
int count = 0;
for (Student student : students) {
if (student.className.equals(className)) {
mathTotalScore += student.mathScore;
englishTotalScore += student.englishScore;
chineseTotalScore += student.chineseScore;
count++;
}
}
System.out.println("班级:" + className);
System.out.println("数学总分:" + mathTotalScore);
System.out.println("英语总分:" + englishTotalScore);
System.out.println("语文总分:" + chineseTotalScore);
System.out.println("学生人数:" + count);
break;
case 2:
System.out.println("请选择学科:");
System.out.println("1. 数学");
System.out.println("2. 英语");
System.out.println("3. 语文");
int subjectChoice = scanner.nextInt();
switch (subjectChoice) {
case 1:
int mathScoreTotal = 0;
for (Student student : students) {
mathScoreTotal += student.mathScore;
}
System.out.println("数学总分:" + mathScoreTotal);
break;
case 2:
int englishScoreTotal = 0;
for (Student student : students) {
englishScoreTotal += student.englishScore;
}
System.out.println("英语总分:" + englishScoreTotal);
break;
case 3:
int chineseScoreTotal = 0;
for (Student student : students) {
chineseScoreTotal += student.chineseScore;
}
System.out.println("语文总分:" + chineseScoreTotal);
break;
default:
System.out.println("无效操作,请重新选择!");
break;
}
break;
default:
System.out.println("无效操作,请重新选择!");
break;
}
}
// 分析学生成绩
static void analyzeStudentScore() {
int mathScoreTotal = 0;
int englishScoreTotal = 0;
int chineseScoreTotal = 0;
int count = 0;
int maxMathScore = Integer.MIN_VALUE;
int maxEnglishScore = Integer.MIN_VALUE;
int maxChineseScore = Integer.MIN_VALUE;
int minMathScore = Integer.MAX_VALUE;
int minEnglishScore = Integer.MAX_VALUE;
int minChineseScore = Integer.MAX_VALUE;
for (Student student : students) {
mathScoreTotal += student.mathScore;
englishScoreTotal += student.englishScore;
chineseScoreTotal += student.chineseScore;
if (student.mathScore > maxMathScore) {
maxMathScore = student.mathScore;
}
if (student.englishScore > maxEnglishScore) {
maxEnglishScore = student.englishScore;
}
if (student.chineseScore > maxChineseScore) {
maxChineseScore = student.chineseScore;
}
if (student.mathScore < minMathScore) {
minMathScore = student.mathScore;
}
if (student.englishScore < minEnglishScore) {
minEnglishScore = student.englishScore;
}
if (student.chineseScore < minChineseScore) {
minChineseScore = student.chineseScore;
}
count++;
}
double mathScoreAvg = (double) mathScoreTotal / count;
double englishScoreAvg = (double) englishScoreTotal / count;
double chineseScoreAvg = (double) chineseScoreTotal / count;
System.out.println("学生数:" + count);
System.out.println("数学平均分:" + mathScoreAvg);
System.out.println("英语平均分:" + englishScoreAvg);
System.out.println("语文平均分:" + chineseScoreAvg);
System.out.println("数学最高分:" + maxMathScore);
System.out.println("英语最高分:" + maxEnglishScore);
System.out.println("语文最高分:" + maxChineseScore);
System.out.println("数学最低分:" + minMathScore);
System.out.println("英语最低分:" + minEnglishScore);
System.out.println("语文最低分:" + minChineseScore);
}
}
```
这个示例程序实现了基本的学生信息录入、查询、统计和分析功能。你可以根据自己的需求,进行修改和完善。
阅读全文
相关推荐
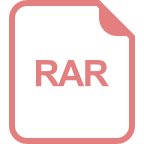
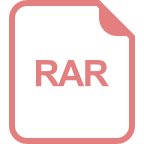
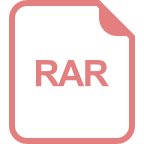
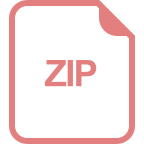
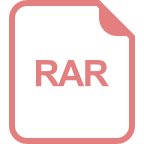
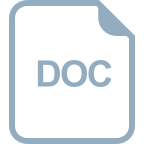
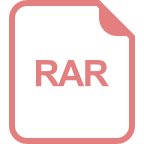
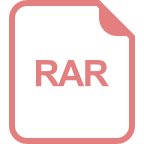
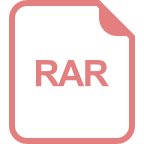
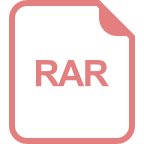
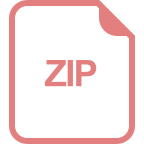
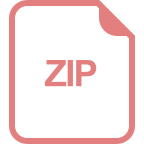
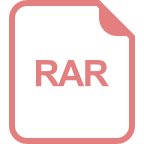
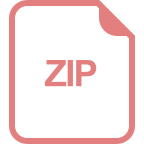