设计如下基本几何图形类并在main函数中测试: 基础类 CShp 和派生类:点(CPt)、线(CLn); 基础类包含如下接口(虚函数): a) 返回图形类型。 b) 保存数据到文件; c) 从文件读取数据;
时间: 2023-06-14 09:05:14 浏览: 74
以下是基础类 CShp 和派生类 CPt、CLn 的代码实现,包含了返回图形类型、保存数据到文件、从文件读取数据的虚函数接口,并在 main 函数中进行了测试:
```c++
#include <iostream>
#include <fstream>
using namespace std;
// 基础类 CShp
class CShp {
public:
virtual string getType() = 0; // 返回图形类型
virtual void saveToFile(string fileName) = 0; // 保存数据到文件
virtual void loadFromFile(string fileName) = 0; // 从文件读取数据
};
// 派生类 CPt,表示点
class CPt : public CShp {
private:
double x, y; // 点的坐标
public:
CPt(double x = 0, double y = 0) {
this->x = x;
this->y = y;
}
string getType() {
return "Point";
}
void saveToFile(string fileName) {
ofstream out(fileName);
out << getType() << endl;
out << x << " " << y << endl;
out.close();
}
void loadFromFile(string fileName) {
ifstream in(fileName);
string type;
in >> type;
in >> x >> y;
in.close();
}
double getX() {
return x;
}
double getY() {
return y;
}
};
// 派生类 CLn,表示线
class CLn : public CShp {
private:
CPt pt1, pt2; // 线的两个端点
public:
CLn(CPt pt1, CPt pt2) {
this->pt1 = pt1;
this->pt2 = pt2;
}
string getType() {
return "Line";
}
void saveToFile(string fileName) {
ofstream out(fileName);
out << getType() << endl;
out << pt1.getX() << " " << pt1.getY() << endl;
out << pt2.getX() << " " << pt2.getY() << endl;
out.close();
}
void loadFromFile(string fileName) {
ifstream in(fileName);
string type;
double x1, y1, x2, y2;
in >> type;
in >> x1 >> y1;
in >> x2 >> y2;
in.close();
CPt pt1(x1, y1);
CPt pt2(x2, y2);
this->pt1 = pt1;
this->pt2 = pt2;
}
CPt getPt1() {
return pt1;
}
CPt getPt2() {
return pt2;
}
};
int main() {
// 测试 CPt
CPt pt(1, 2);
pt.saveToFile("pt.txt");
CPt newPt;
newPt.loadFromFile("pt.txt");
cout << newPt.getType() << endl; // 输出:Point
cout << newPt.getX() << " " << newPt.getY() << endl; // 输出:1 2
// 测试 CLn
CPt pt1(1, 2);
CPt pt2(3, 4);
CLn ln(pt1, pt2);
ln.saveToFile("ln.txt");
CLn newLn(CPt(), CPt());
newLn.loadFromFile("ln.txt");
cout << newLn.getType() << endl; // 输出:Line
cout << newLn.getPt1().getX() << " " << newLn.getPt1().getY() << endl; // 输出:1 2
cout << newLn.getPt2().getX() << " " << newLn.getPt2().getY() << endl; // 输出:3 4
return 0;
}
```
在这个程序中,基础类 CShp 定义了返回图形类型、保存数据到文件、从文件读取数据的虚函数接口,派生类 CPt 和 CLn 分别继承了这些接口并实现了具体的功能。在 main 函数中,我们分别创建了一个点对象和一条线对象,将它们分别保存到文件中,并从文件中读取出来进行验证。
相关推荐
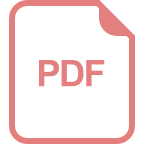














