在C语言中,如何以每行四个输入多行字符串,最后一行以#结尾
时间: 2023-11-28 09:48:45 浏览: 36
你可以使用循环和fgets函数来实现以每行四个输入多行字符串,最后一行以#结尾的功能。具体实现如下:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LINE_LENGTH 1000
#define MAX_LINES 100
int main() {
char lines[MAX_LINES][MAX_LINE_LENGTH];
int line_count = 0;
int line_length;
printf("Please input strings, end with '#' in a single line:\n");
while (line_count < MAX_LINES) {
line_length = 0;
// Read a line of input
fgets(lines[line_count], MAX_LINE_LENGTH, stdin);
// Remove the newline character from the input
lines[line_count][strcspn(lines[line_count], "\n")] = 0;
// Check if the line ends with '#'
if (lines[line_count][strlen(lines[line_count]) - 1] == '#') {
// Replace '#' with '\0' to terminate the string
lines[line_count][strlen(lines[line_count]) - 1] = '\0';
line_count++;
break;
}
// Check if we have read four lines of input
if ((line_count + 1) % 4 == 0) {
line_count++;
break;
}
line_count++;
}
printf("The input strings are:\n");
for (int i = 0; i < line_count; i++) {
printf("%d: %s\n", i + 1, lines[i]);
}
return 0;
}
```
在这个程序中,我们定义了一个二维字符数组 `lines` 来存储输入的多行字符串,每行字符串的最大长度为 `MAX_LINE_LENGTH`,最大行数为 `MAX_LINES`。我们使用一个循环来读取输入的每一行字符串,然后进行处理。
在循环中,我们首先使用 `fgets` 函数读取一行输入,并使用 `strcspn` 函数去掉字符串末尾的换行符。然后我们检查这行字符串是否以 '#' 结尾,如果是,我们将 '#' 替换为 '\0' 来终止字符串,并退出循环。如果不是,我们检查是否已经读取了四行字符串,如果是,我们也退出循环。如果既不是以 '#' 结尾,也没有读取四行字符串,我们继续读取下一行输入。
最后,我们输出所有输入的字符串,并以行号进行编号。
相关推荐
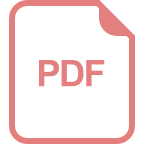
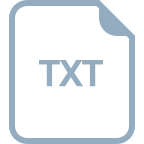
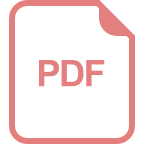














