java 单例模式 三种模式 代码示例
时间: 2023-06-29 18:19:55 浏览: 66
在 Java 中,单例模式一般有以下三种实现方式:
### 1. 懒汉式单例模式
在第一次调用 `getInstance()` 方法时才会创建单例对象。
```java
public class Singleton {
private static Singleton instance;
private Singleton() {
// 防止外部实例化
}
public static synchronized Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
```
### 2. 饿汉式单例模式
在类加载时就创建单例对象。
```java
public class Singleton {
private static Singleton instance = new Singleton();
private Singleton() {
// 防止外部实例化
}
public static Singleton getInstance() {
return instance;
}
}
```
### 3. 双重校验锁单例模式
使用双重校验锁可以避免多线程环境下的线程安全问题,同时也实现了懒加载的效果。
```java
public class Singleton {
private static volatile Singleton instance;
private Singleton() {
// 防止外部实例化
}
public static Singleton getInstance() {
if (instance == null) {
synchronized (Singleton.class) {
if (instance == null) {
instance = new Singleton();
}
}
}
return instance;
}
}
```
在双重校验锁单例模式中,使用 `volatile` 关键字可以保证多线程环境下的可见性和有序性,从而避免出现线程安全问题。同时,使用双重判断可以避免不必要的加锁,提高了代码的执行效率。
阅读全文
相关推荐
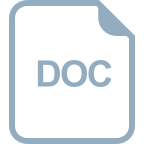
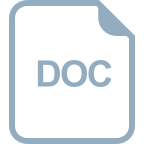
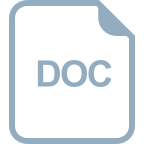
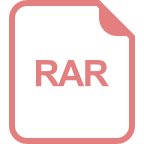




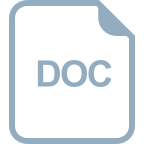
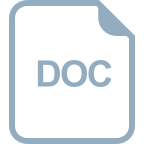
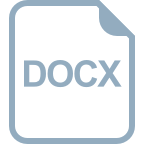
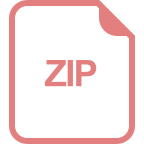