Python实现鸢尾花数据集以下操作:Tensorflow2的数据加载、多分类模型的搭建、分类模型的交叉验证、模型保持和新数据预测。
时间: 2024-05-13 09:14:50 浏览: 89
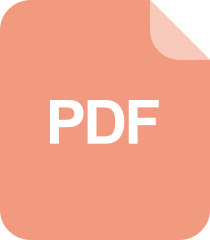
鸢尾花数据集操作指导
好的,我来为您介绍如何实现这些操作。
首先,我们需要导入相关的库:
```python
import tensorflow as tf
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import LabelBinarizer
```
然后,我们可以加载鸢尾花数据集并将其拆分为训练集和测试集:
```python
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2, random_state=42)
```
接下来,我们需要对标签进行独热编码:
```python
encoder = LabelBinarizer()
y_train = encoder.fit_transform(y_train)
y_test = encoder.transform(y_test)
```
现在,我们可以使用TensorFlow2构建一个多分类模型:
```python
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(4,)),
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(3, activation='softmax')
])
```
我们使用两个Dense层来构建一个具有64个神经元的隐藏层,使用ReLU激活函数。最后一层是一个具有3个输出的softmax层,用于多分类。
现在,我们可以编译模型并使用交叉验证拟合:
```python
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
history = model.fit(X_train, y_train, epochs=50, validation_split=0.2)
```
我们使用Adam优化器和交叉熵损失函数来编译模型,并使用80%的数据进行交叉验证。
最后,我们可以使用测试集来评估模型:
```python
test_loss, test_acc = model.evaluate(X_test, y_test)
print('Test accuracy:', test_acc)
```
如果我们想要保存模型以供以后使用,可以使用以下代码:
```python
model.save('iris_model.h5')
```
最后,我们可以使用新数据对模型进行预测:
```python
import numpy as np
new_data = np.array([[5.1, 3.5, 1.4, 0.2], [6.2, 3.4, 5.4, 2.3], [7.7, 2.6, 6.9, 2.3]])
predictions = model.predict(new_data)
print(predictions)
```
这将输出模型对新数据的预测概率。
阅读全文
相关推荐
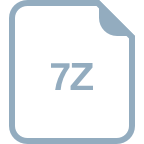
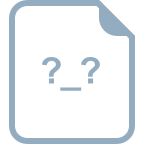
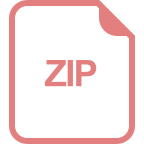
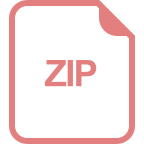
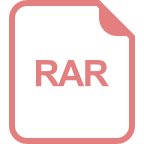
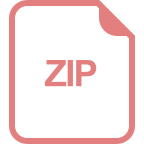
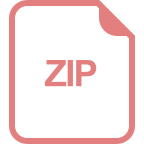
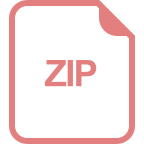
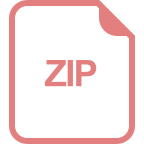
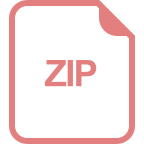
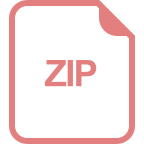
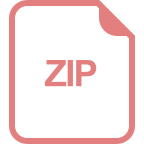
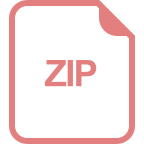
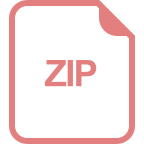
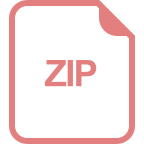