const res = await db.collection('usersproducts').aggregate() .match({ [_.in(event.query.productOpenid)]: _.exists(true) }) .sample({ size: 20 }) .end() ps = res.list这段代码会报错,报错信息是:Error: cloud.callFunction:fail Error: errCode: -504002 functions execute fail | errMsg: TypeError: Cannot read property 'productOpenid' of undefined。你能否把这个问题修复,并把正确的代码发给我
时间: 2023-06-17 07:07:26 浏览: 77
这个错误提示表明 `event.query` 是 `undefined`,因此 `event.query.productOpenid` 会抛出 `TypeError: Cannot read property 'productOpenid' of undefined` 的异常。你需要确保传入的 `event` 参数包含了 `query` 属性并且 `query` 属性包含了 `productOpenid` 字段。
以下是可能的解决方案:
1. 检查调用云函数的代码,确保传入了正确的参数。
2. 在云函数中添加参数校验,防止未传入必要的参数。
3. 检查数据库中是否存在符合条件的数据,如果不存在,可能会导致查询失败。
另外,你的代码中使用了 `_.in` 和 `_.exists` 这两个函数,这可能是 `underscore` 库的函数。请确保已经正确引用了 `underscore` 库,并且 `_.in` 和 `_.exists` 函数能够正常使用。
下面是修改后的代码,你可以把它作为参考:
```
const res = await db.collection('usersproducts')
.aggregate()
.match({
productOpenid: _.in([event.query.productOpenid]),
productName: _.exists(true)
})
.sample({
size: 20
})
.end()
const ps = res.list
```
相关问题
微信小程序云开发云函数res = await db.collection('usersproducts').where({ _openid: _.in(event.sampleid.productOpenid) }) .aggregate() .sample({ size: event.size }) .end() ps = res.list这段代码报错,报错信息是:Error: cloud.callFunction:fail Error: errCode: -504002 functions execute fail | errMsg: TypeError: db.collection(...).where(...).aggregate is not a function
这个错误提示是因为 `aggregate` 函数不支持在云函数中使用,它是 MongoDB 数据库中的聚合函数。在小程序云开发中,可以使用 `get()` 或 `field()` 函数来实现类似的功能。
你可以尝试将代码修改为以下形式:
```
const db = wx.cloud.database()
const _ = db.command
const res = await db.collection('usersproducts')
.where({
_openid: _.in(event.sampleid.productOpenid)
})
.field({
// 如果需要随机获取数据,可以添加一个随机字段
random: db.command.rand(),
// 返回结果中只需要 _id 字段
_id: true
})
.get()
const ps = res.data
```
这样就可以实现在云函数中根据指定的 _openid 随机获取指定数量的数据了。
const countResult = await db.collection('request_info').count()
这是一个使用 MongoDB 数据库的 Node.js 代码片段。它使用了 MongoDB 的 Node.js 驱动程序中的集合对象的 count() 方法,来获取 "request_info" 集合中的文档数量,并将结果保存在一个名为 "countResult" 的变量中。这个代码片段在使用 await 语法来等待 count() 方法的返回结果。
相关推荐
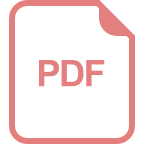
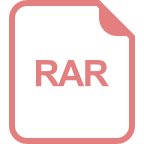












