使用浏览器提供的localStorage或IndexedDB存储一个随机生成的唯一标识符,每次访问网站时从存储中读取。
时间: 2024-03-26 17:38:01 浏览: 97
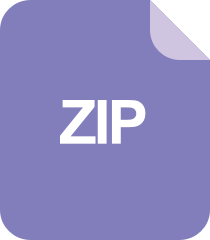
Redux-LocalStorage:在浏览器本地存储中存储状态
是的,这是一种获取浏览器不变的唯一标识符的方法。具体实现可以参考以下代码:
```javascript
// 生成一个随机的唯一标识符
function generateUUID() {
var d = new Date().getTime();
var uuid = 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) {
var r = (d + Math.random() * 16) % 16 | 0;
d = Math.floor(d / 16);
return (c == 'x' ? r : (r & 0x3 | 0x8)).toString(16);
});
return uuid;
}
// 检查浏览器是否支持localStorage或IndexedDB
function isLocalStorageSupported() {
try {
var testKey = 'test';
var storage = window.localStorage;
storage.setItem(testKey, '1');
storage.removeItem(testKey);
return true;
} catch (error) {
return false;
}
}
function isIndexedDBSupported() {
return 'indexedDB' in window;
}
// 从localStorage或IndexedDB中读取唯一标识符
function getUniqueID() {
var uniqueID = '';
if (isLocalStorageSupported()) {
var storage = window.localStorage;
uniqueID = storage.getItem('uniqueID');
if (!uniqueID) {
uniqueID = generateUUID();
storage.setItem('uniqueID', uniqueID);
}
} else if (isIndexedDBSupported()) {
var request = window.indexedDB.open('uniqueID', 1);
request.onerror = function(event) {
console.log('Failed to open indexedDB');
};
request.onupgradeneeded = function(event) {
var db = event.target.result;
var objectStore = db.createObjectStore('uniqueID', { keyPath: 'id' });
var uniqueID = generateUUID();
objectStore.add({ id: 1, value: uniqueID });
};
request.onsuccess = function(event) {
var db = event.target.result;
var transaction = db.transaction(['uniqueID'], 'readwrite');
var objectStore = transaction.objectStore('uniqueID');
var getRequest = objectStore.get(1);
getRequest.onsuccess = function(event) {
if (getRequest.result) {
uniqueID = getRequest.result.value;
} else {
uniqueID = generateUUID();
objectStore.add({ id: 1, value: uniqueID });
}
};
};
} else {
uniqueID = generateUUID();
}
return uniqueID;
}
```
以上代码中,`generateUUID()`函数用于生成一个随机的唯一标识符,`isLocalStorageSupported()`和`isIndexedDBSupported()`函数用于检查浏览器是否支持localStorage或IndexedDB,`getUniqueID()`函数用于从localStorage或IndexedDB中读取唯一标识符,如果没有则生成一个新的唯一标识符并存储到localStorage或IndexedDB中。
阅读全文
相关推荐
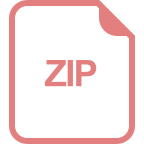
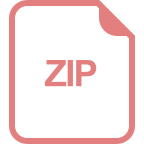
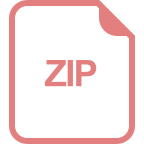
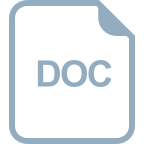
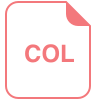
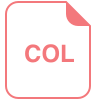
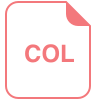
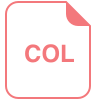
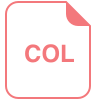
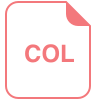
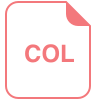

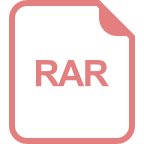
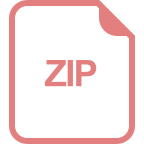
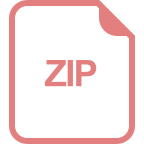